The f-string expression part cannot include a backslash, a type of SyntaxError that often occurs when a backlash is used between the curly braces of a formatted string. This error can be complex to resolve. This guide will give you a walkthrough about the f-string expression part that cannot include a backslash SyntaxError and ways to resolve it.
Contents
What is the f-string expression part that cannot include a backslash?
The f-string expression part cannot include a backslash, a type of SyntaxError. This error occurs when a backlash is present in the curly brackets of an f-string, and you try to contain the escape characters.
This error can be observed when two separate string variables are tried to print by using the f-string format and joined together by an escape character, which is taken in between the curly brackets.
Syntax: –
first_name = "Nathan"
last_name = "Sebhastian"
print(f"{first_name + '\t' + last_name}")
The above code will show the f-string expression part cannot include a backslash SyntaxError as there is an escape character and a backlash is present in between the curly brackets.
How do we resolve the f-string expression part cannot include a backslash?
The f-string expression part cannot include a backslash. SyntaxError can be resolved quickly by following the solutions mentioned below.
Adding the Backlash at the beginning
One of the easiest ways to resolve the f-string expression part that cannot include a backslash SyntaxError is to move out the Backlash to the beginning of the string. Removing the Backlash between the strings and adding them at the beginning of the string will not affect the result and will remain unchanged.
Syntax: –
employees = ['John', 'Jane', 'Wayne']
newline_char = '\n'
my_str = f'Employees list: \n{newline_char.join(employees)}'
print(my_str)
The above code will show the result as the one with the Backlash present in between the strings.
Converting the Backlash into variable
Another way to resolve the f-string expression part that cannot include a backslash SyntaxError is to convert the Backlash in the string to a variable. It can only be done by extracting the Backlash into a variable and adding it to the variables in the string.
Syntax: –
backslash = '\\'
first = 'John'
last = 'Smith'
a_str = f'{backslash} {first} {backslash} {last}'
print(a_str)
Replacing the \n Backlash with other functions
Another way to resolve the f-string expression part that cannot include a backslash SyntaxError is to replace the Backlash with the other line separating functions like os.linesep and join(). It is usually used in place of the \n escape element to add a new line between the string’s contents.
Syntax for os.linesep: –
import os
my_str = f"John{os.linesep}Smith"
print(my_str)
Syntax for join(): -
first_name = "Nathan"
last_name = "Sebhastian"
print(f"{first_name + backslash + last_name}")
This will result in a similar way as the \n escape element is supposed to.
Using chr() function
Another way to resolve the f-string expression part that cannot include a backslash SyntaxError is to use the chr() function to add a backlash into the string without raising the error. The chr() function returns the characters representing the specified Unicode. In this function, a specific number represents a valid and specified Unicode.
Syntax; –
first = 'John'
last = 'Smith'
a_str = f'{chr(10)} {first} {chr(10)} {last}'
print(a_str)
Using str.format() function
Another method to resolve this error is to use str.format() function. In this method the str.format() function will return the argument without raising any error.
Syntax:
import os
# First part
my_str = f"John\nSmith"
print(my_str)
# Second part
first_name = "Nathan"
last_name = "Sebhastian"
formatted_name = "{}{}".format(first_name, last_name)
print(formatted_name)
FAQs
What is a f-string?
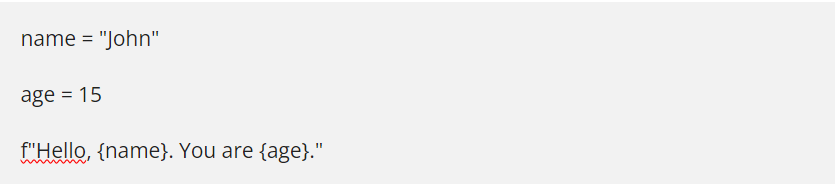
The f-string in Python, also known as the formatted string literals are string literals with curly braces with expressions that can be replaced with a value and a f at the beginning of the string.
Syntax: –
What is \t Backlash?

The Backlash is a unique character in Python strings that separate a string’s contents. It is also known as the escape character. Based on the gap required, it can be of three types.
For example, \t adds a tab gap between the string contents.
Syntax: –
What is \n Backlash?

Another type of escape character is \n. This escape character is used when a new line must be added between the contents of a string.
Syntax: –
What is \r Backlash?

The last type of escape character is \r, which adds a carriage return. It takes the contents after the \r in the string to the beginning of the line and keeps replacing the characters until all the contents after \r are placed at the beginning.
Syntax: –
What is an os.linesep?

The os.linesep in Python is a separator used to indicate the characters used by the operating system to end the lines. It can be used in place of escape elements like \n and \r.
Syntax: –
Conclusion
This guide has all the information that can help you understand and find the causes for the f-string expression part that cannot include a backslash SyntaxError and the probable solutions that can help you resolve the error without any issues.
References
Follow us at PythonClear to learn more about solutions to general errors one may encounter while programming in Python.