This article will discuss a common error that occurs when using the plt.savefig function in Python. This function saves a matplotlib figure as an image file, such as PNG, JPG, or PDF.
However, ,the saved image file is sometimes blank or empty, even though the figure is displayed correctly on the screen. This can be frustrating and confusing while saving the plots for further analysis or presentation. Here, we will give you a walkthrough about the error, how to avoid it, and probable solutions.
Contents
What is the “plt.savefig blank” error?
The “plt.savefig blank” error is when the plt.savefig function does not save the figure properly and produces a blank or empty image file. This means the image file does not contain any data or pixels representing the figure.
The file size may be very small or zero, and any image viewer or editor may not open the file. The plt.savefig blank” error can occur for various reasons, such as incorrect file format, wrong file path, incompatible backend, or incorrect order of commands.
What causes the “plt.savefig blank” error?
There are several possible causes for the “plt.savefig blank” error, depending on the specific situation and the code used. Here are some of the common causes and how to identify them:
Incorrect file format
One of the possible causes is that the file format specified in the plt.savefig function is not supported by matplotlib or the backend is used. For example, if you try to save a figure as a GIF file, you may get a blank image file because matplotlib does not support GIF format by default.
To check if the file format is supported, use the plt.gcf().canvas.get_supported_filetypes() command returns a dictionary of supported file formats and their extensions. For example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
plt.gcf().canvas.get_supported_filetypes()
This will output something like:
{'eps': 'Encapsulated Postscript',
'jpeg': 'Joint Photographic Experts Group',
'jpg': 'Joint Photographic Experts Group',
'pdf': 'Portable Document Format',
'pgf': 'PGF code for LaTeX',
'png': 'Portable Network Graphics',
'ps': 'Postscript',
'raw': 'Raw RGBA bitmap',
'rgba': 'Raw RGBA bitmap',
'svg': 'Scalable Vector Graphics',
'svgz': 'Scalable Vector Graphics',
'tif': 'Tagged Image File Format',
'tiff': 'Tagged Image File Format'}
If the file format you want to use is not in the dictionary, you may need to install additional libraries or change the backend to support it.
Wrong file path
Another possible cause is that the file path specified in the plt.savefig function is not valid or accessible. For example, you may get a blank image file if you try to save a figure to a folder that does not exist or to a file that contains illegal characters.
To verify if the file path is valid, you can use the os.path.exists function, which returns True if the path exists and False otherwise. For example:
import os
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
os.path.exists("C:/Users/username/Desktop/myplot.png")
This will output True if the file path is valid and False otherwise. If the file path is invalid, you may need to create the folder, change the file name, or use a different location to save the figure.
Incompatible backend
Another possible cause is that the backend used by matplotlib is not compatible with the file format or the operating system you are using. The backend is the engine that renders the figure on the screen or the file. Matplotlib supports various backends, such as TkAgg, Qt5Agg, GTK3Agg, etc.
Some backends may not support certain file formats or have issues with certain operating systems. To check the current backend, you can use the matplotlib.get_backend() function, which returns the name of the backend.
For example:
import matplotlib
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
matplotlib.get_backend()
This will output something like:
'TkAgg'
If the backend is not compatible with the file format or the operating system you are using, you may need to change the backend to a different one. You can do this using the matplotlib.use the function which sets the backend to the one you specify. For example:
import matplotlib
matplotlib.use('Qt5Agg')
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
plt.savefig('myplot.png')
This will use the Qt5Agg backend to save the figure as a PNG file. Note that you need to call the matplotlib.use a function before importing matplotlib.pyplot; otherwise, it will have no effect.
How do we resolve the “plt.savefig blank” error?
To resolve the “plt.savefig blank” error, the first step is to identify the cause of the error and apply the appropriate solution. The cause of the error can be one of the following:
- The file format you are using is not supported by matplotlib or the backend you are using. In this case, you must change the file format or the backend to a compatible one.
- The file path that you are using is not valid or accessible. In this case, you must create the folder, change the file name, or use a different location to save the figure.
- The order of the commands that you are using is incorrect. In this case, you must save the figure after creating and closing it.
- The backend you are using is not compatible with the file format or operating system you are using. In this case, you must change the backend to a different one.
If the above solutions do not work, you can try to use some additional arguments in the plt.savefig function, such as dpi, bbox_inches, or transparent. These arguments can affect the saved image file’s quality, size, and appearance.
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
# Save the figure with a higher resolution, a tight bounding box, and a transparent background
plt.savefig('myplot.png', dpi=300, bbox_inches='tight', transparent=True)
This will save the figure as a PNG file with a higher resolution, a tight bounding box, and a transparent background.
This will give the following graph:
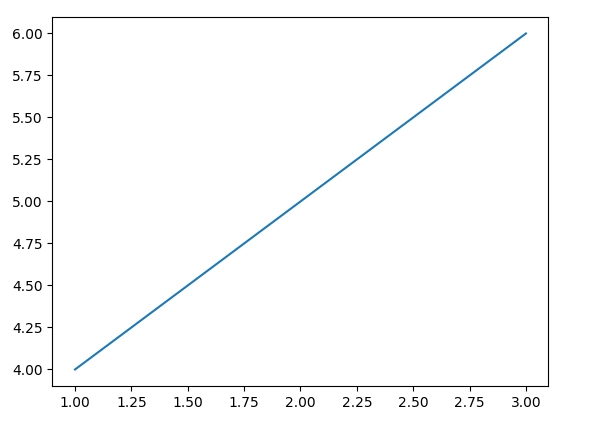
Use Correct order of commands
Another possible cause is incorrect order of the commands used to create and save the figure. For example, you may get a blank image file if you try to save the figure before creating it or after closing it. To avoid this, you need to follow the correct order of commands, which is:
- Create the figure and the axes using plt.figure and plt.subplot (optional)
- Plot the data using plt.plot, plt.scatter, plt.bar, etc.
- Customize the figure using plt.title, plt.xlabel, plt.ylabel, etc.
- Save the figure using plt.savefig
- Show the figure using plt.show (optional)
- Close the figure using plt.close (optional)
For example:
import matplotlib.pyplot as plt
# Create the figure and the axes
fig, ax = plt.subplots(figsize=(10, 6))
# Plot the data
ax.plot([1, 2, 3], [4, 5, 6], label='Line')
ax.scatter([1, 2, 3], [7, 8, 9], label='Scatter')
# Customize the figure
ax.set_title('My Plot')
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.legend()
# Save the figure
plt.savefig('myplot.png')
# Show the figure
plt.show()
# Close the figure
plt.close()
This will create and save a figure like this:
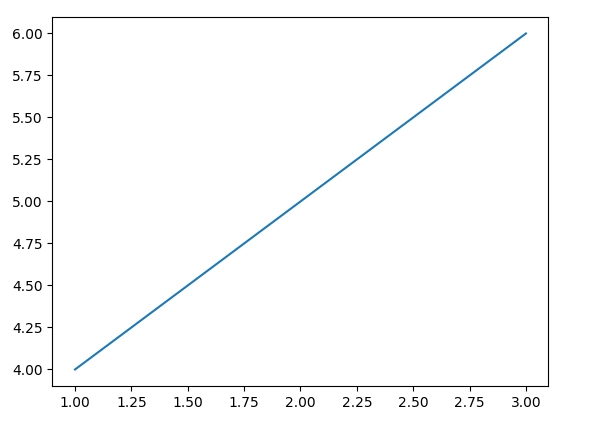
FAQs
How can I save multiple figures in one file?
You can use the PdfPages class from the matplotlib.backends.backend_pdf module, which allows you to create a PDF file and append multiple figures.
This will create a PDF file with two figures in it.
How can I save a figure without displaying it on the screen?
You can use the plt.off function, which turns off the interactive mode of matplotlib.
This will create and save a figure with a specific size and aspect ratio.
How can I save a figure with a specific size and aspect ratio?
You can use the figsize and dpi arguments in the plt.figure function, which sets the size and resolution of the figure in inches and dots per inch, respectively. You can also use the aspect argument in the plt.axes function, which sets the aspect ratio of the axes. For example:
This will create and save a figure with a specific size and aspect ratio.
How can I save a figure with a transparent background?
You can use the transparent argument in the plt.savefig function, which sets the figure’s background to be transparent. By default, this argument is False, meaning the background is white. If you set it to True, the background will be transparent. For example:
This will save the figure with a transparent background.
Conclusion
In this article, we have discussed the “plt.savefig blank” error, which occurs when the plt.savefig function does not save the matplotlib figure properly and produces a blank or empty image file. We have explained the possible causes of this error, such as incorrect file format, wrong file path, incompatible backend, or incorrect order of commands.
We have also provided some steps to resolve this error, such as checking the file format and path, changing the backend, or using additional arguments in the plt.savefig function. We hope this article has helped you understand and fix this error and successfully save your plots.
References
To learn more about some common errors follow Python Clear’s errors section.