Syntaxerror: multiple statements found while compiling a single statement is a common error and is straightforward to fix. This error is pretty self-explanatory and occurs due to the presence of multiple statements in a code where it can only support a single statement.
This guide will help you find the cause of the error and resolve it.
Contents
- 1 What is SyntaxError?
- 2 How to fix SyntaxError?
- 3 What is compiling?
- 4 What is SyntaxError: multiple statements found while compiling a single statement?
- 5 Causes for the SyntaxError: multiple statements found while compiling a single statement
- 6 Solution for SyntaxError: multiple statements found while compiling a single statement Error
- 7 FAQ
- 8 Conclusion
- 9 References
What is SyntaxError?
While working in Python, facing SyntaxError is common. This error occurs when the source is translated to byte code. It is generally related to minute details while writing the code. The SyntaxError message pops up when you emmit certain symbols or misspell certain functions.
How to fix SyntaxError?
Unlike exception errors, this type of error is easy to resolve after you find the source of the error. Finding the basis of the error takes a few steps, as errors can not be located from the message.
Simply put, you can correct the errors by updating misspelled reserved words, using misused block statements, adding missing required spaces, missing quotes, missing assignment operators, etc.
Sometimes this error may be caused by the invalid function calling or defining or invalid variable declaration, which you can resolve by putting appropriate functions.
What is compiling?
Compiling translates the understandable human code to machine code so that the CPU can directly execute the instructions. In Python, the compile() function is used to convert the source code to a code object which can later be performed by the exec() function.
What is SyntaxError: multiple statements found while compiling a single statement?
The syntaxerror: multiple statements found while compiling a single statement is a SyntaxError that arises when multiple statements are being declared in a script set to be executed. This is because, in an interactive interpreter, compiling more than one statement at a time is not allowed.
Causes for the SyntaxError: multiple statements found while compiling a single statement
As mentioned above, this type of error is the presence of multiple statements in a script when the interactive interpreter only allows a single statement and would not proceed with compiling.
Syntax:-
k = 2
k += 2
print(k)
The above code will return you with the syntaxerror: multiple statements found while compiling a single statement error message.
>>> k = 1
k += 1
print(k)
File "<stdin>", line 1
k += 1
print(k)
^
SyntaxError: multiple statements found while compiling a single statement
>>>
Solution for SyntaxError: multiple statements found while compiling a single statement Error
The SyntaxError: multiple statements found while compiling a single statement error can be resolved by simply deleting extra statements and proceeding with only a single statement. This error can be resolved in many ways, such as:
Compile one statement at a time
Instead of trying to compile many statements at once, you can try to compile one statement at a time. This one might seem a long process, but it is undoubtedly a better method to resolve the SyntaxError: multiple statements found while compiling a single statement error.
Syntax:
>>> a = 1
b = 2
This will generate the SyntaxError: multiple statements found while compiling a single statement error. So instead, you can try:
>>>a = 1
>>>b = 2
>>>
Separating statements
Another reason for this type of error can be the usage of an interactive shell. This type of shell only allows one statement at a time. So to avoid the SyntaxError: multiple statements found while compiling a single statement error, you can try to use semicolons in between every line.
Syntax:
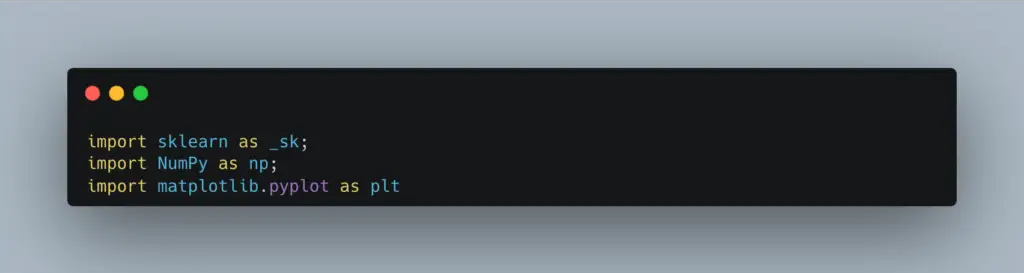
Apart from this, you can also try to create a new file by control+n and save it before running by. This way, you can find the normal idle.
Using the throw-away function
Another way of resolving the SyntaxError: multiple statements found while compiling a single statement error is to put things into a throw-away function. Here you can use the def abc() function. This will help you in pasting the supplementary statement and compiling it. This method might be a quick solution, but it won’t work every time.
Syntax:
def abc():
k = 1
k += 1
print(k)
This will work and will return the output without showing the error message.
def abc():
k = 1
k += 1
print(k)
abc()
2
Using other GUI (Graphical User Interface)
This method is best for a long-term solution. Changing the GUI (Graphical User Interface) for running Python will help to resolve the SyntaxError: multiple statements found while compiling a single statement error in the long term. Here you can use other GUI such as IDLE anycodings_python or M-x run-python in Emacs.
FAQ
What’s the difference between single and multiple statements while compiling?
When you compile a single statement, you only include a single script and compile it, which does not raise any issue if done correctly. But on the other hand, when you compile multiple statements, you take multiple statements in a single script which may raise the SyntaxError: multiple statements found while compiling a single statement error which you can resolve by using the above solution list.
Conclusion
This article will help you find the cause for the SyntaxError: multiple statements found while compiling a single statement error and will help you with its solution.
References
Also, follow pythonclear/errors to learn more about error handling.