We are often required to prepend lists in python, where we need to add an element at the beginning of the list.
Contents
1. What does it mean to prepend lists?
To prepend so something means to add something to the beginning of something else. In python, a list is an object with an inbuilt library of functions like append(), extend(), index(), insert(), pop(), reverse (), sort(),etc. Lists are very versatile objects and can be used for solving a large variety of problems. We often use the append() function to add an element at the end of a list. But sometimes we may encounter a problem where we need to add an element at the beginning of a list i.e, to prepend lists.
Let’s say we have a list
A = [2, 3, 4]
Here list A contains elements 2,3 and 4 and you want to add element 1 to the beginning of list A. Your desired output should look like this:
A = [1, 2, 3, 4]
Prepending a list can be useful in solving several problems. For example, we have a log of events in a python list and we want to add the most recent event at the top of the list.
There are several ways one can prepend lists.
1.1 A naive way to prepend list
The additive nature of lists is an important feature and property of lists. One can exploit this property to prepend lists in a very straightforward way.
In this method, let’s assume we have a list A containing elements 2,3, and 4 and you want to add element 1 to the list. Make another list B containing only one element 1 and add list A to B, which will result in a list with element 1 at the beginning followed by elements of list A. You can follow the code below:
A = [2, 3, 4]
B = [1]
C = B+A
print(C)
Output:
[1, 2, 3, 4]
This is a simple and easy way to prepend lists, although not very efficient and professional.
1.2 Using deque to prepend list
In this approach, you can use appendleft() function of a deque (double-ended queue) from the collections library. So first we need to convert the list to a deque object and then use function appendleft() to push the elements by one space (i.e, to make space at the front of the deque) and add a new element at the front.
from collections import deque
A = [2, 3, 4]
A = deque(A)
A.appendleft(1)
print(A)
Output:
deque([1, 2, 3, 4])
You can convert the deque back to a list by using the list() function as follows:
from collections import deque
A = [2, 3, 4]
A = deque(A)
A.appendleft(1)
A = list(A)
print(A)
Output:
[1, 2, 3, 4]
To learn more about deque go to collections documentation.
1.3 Using list.insert() function to prepend list
insert() is an inbuilt list library function that inserts an element in the list at the specified index. Function insert() takes in 2 arguments, first the index you want to insert an element and, second the element itself. Hence to prepend an element to the list we need to insert out element at the 0th index. Example:
A = [2,3,4]
A.insert(0,1)
print(A)
Output:
[1, 2, 3, 4]
This is the most efficient and prevalent method to prepend a list.
1.4 Using reverse-append-reverse technique to prepend list
This technique is neither efficient nor straightforward. In this technique, you first reverse the list elements by using the reverse() function then append the element we wanted to add at the front of the list, then reverse the list again to get the desired list.
A = [2, 3, 4]
A.reverse()
A.append(1)
A.reverse()
print(A)
Output:
[1, 2, 3, 4]
1.5 Prepend list by sorting
This technique can be used only in specific conditions like in the example we are following so far with A =[2,3,4] we can append 1 and sort the list using the sort() function. Also can be used to maintain logs where each element carries a time stamp, and one can sort the list according to the time stamp after appending a new element.
A = [2, 3, 4]
A.append(1)
A.sort()
print(A)
Output:
[1, 2, 3, 4]
An example where time-stamped logs need to be prepended.
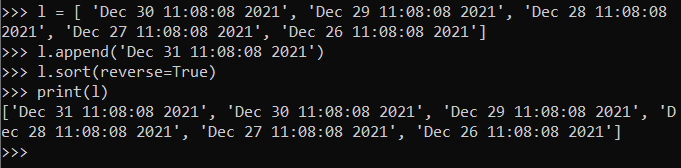
2. Prepend string to list in Python
To prepend lists with string one can follow similar methods as mentioned above. The easiest way to do this is to use the insert() function as explained in 1.3. For example
A = ['banana', 'orange', 'mango']
A.insert(0,'apple')
print(A)
Output:
['apple', 'banana', 'orange', 'mango']
The naive method explained in section 1.1 can also be used to prepend lists with string.
A = ['banana', 'orange', 'mango']
A = ['apple'] +A
print(A)
Output
['apple', 'banana', 'orange', 'mango']
3. Prepend none to a list Python
We can prepend lists with None by adding any of the first four methods explained above. For example, using deque,
from collections import deque
A = [1, 2, 3, 4]
A = deque(A)
A.appendleft(None)
A = list(A)
print(A)
Output:
[None, 1, 2, 3, 4]
Using insert() function to prepend lists with None.
A = [1, 2, 3, 4]
A.insert(0,None)
print(A)
Output:
[None, 1, 2, 3, 4]
4. Prepend string to elements in a list
Unlike the topic of discussion in this article, this problem involves prepending strings to elements in the list. Similar to lists strings can also be appended or prepended in Python. To prepend a string to each element in a list one can either use the additive property of strings or can used f-strings
4.1 Using Additive property
One can add the string that is to be prepended to the element in the list with a for loop. For example, to prepend a string ‘string’ to a list of integers.
A = [1, 2, 3, 4]
B = ["string" + str(x) for x in a]
print(B)
Output:
['string1', 'string2', 'string3', 'string3']
4.2 Using f-strings
With the help of format strings, the solution to this problem becomes very simple. One can write a variable in {} in an f-string, that variable will then be converted to a string and inserted at the {} position in the f-string. This method can effectively solve this problem to prepend a string to elements in a list.
A = [1, 2, 3, 4]
B = [f'string{x}' for x in a]
print(B)
Output:
['string1', 'string2', 'string3', 'string3']
That’s it for this post. Keep reading Python Clear to gain more Python Knowledge!