In the vast world of programming languages Python is one such language used for various purposes, like web development, machine learning, data science, and many more. But like any other programming language, Python is not perfect, and sometimes you may encounter errors or problems when working with it. One such error is the Error: must request at least one color from a hue palette.
This article will give you a walkthrough about the causes of this error and ways to resolve it using the ggplot2 package. We will also show you how to customize the plots’ color, size, shape, and labels using different functions and arguments. By this article’s end, you can create beautiful and informative plots in Python without encountering this error. Let’s get started!
Contents
What is the “Error: must request at least one colour from a hue palette.” error?
This error occurs when using the ggplot2 package, a powerful and elegant tool for creating plots and graphics in Python. The ggplot2 is based on the grammar of graphics, a system for describing and building plots using layers of components. One of these components is the aes function, which maps variables to aesthetic attributes such as color, shape, size, etc.
The error message indicates something is wrong with the color mapping in the aes function. Specifically, the value assigned to the col or fill argument is not a valid color or a factor variable. A factor variable is categorical with a finite set of levels, such as gender, species, or country. A hue palette is a set of colors assigned to each level of a factor variable and can be customized using functions such as scale_color_hue or scale_fill_hue.
What causes the “Error: must request at least one colour from a hue palette.”?
The most common cause of this error is when the col or fill argument inside the aes function is assigned a value that is not a valid color or a factor variable. A factor variable is categorical with a finite set of levels, such as gender, species, or country. A hue palette is a set of colors assigned to each level of a factor variable and can be customized using functions such as scale_color_hue or scale_fill_hue.
Invalid color
We will get the error if we assign a value that is not a valid color name or code to the col or fill argument, such as “NA” or “foo”. For example, suppose we have a data frame called df that contains the following columns:
- x: a numeric variable
- y: a numeric variable
- z: a factor variable with two levels: “A” and “B”
If we want to create a scatter plot of x and y and color the points by z, we can use the following code:
import pandas as pd
import plotnine as p9
df = pd.DataFrame({
"x": [1, 2, 3, 4],
"y": [5, 6, 7, 8],
"z": ["A", "B", "A", "B"]
})
(p9.ggplot(df, p9.aes(x="x", y="y", col="z"))
+ p9.geom_point())
This code will produce a plot like this:
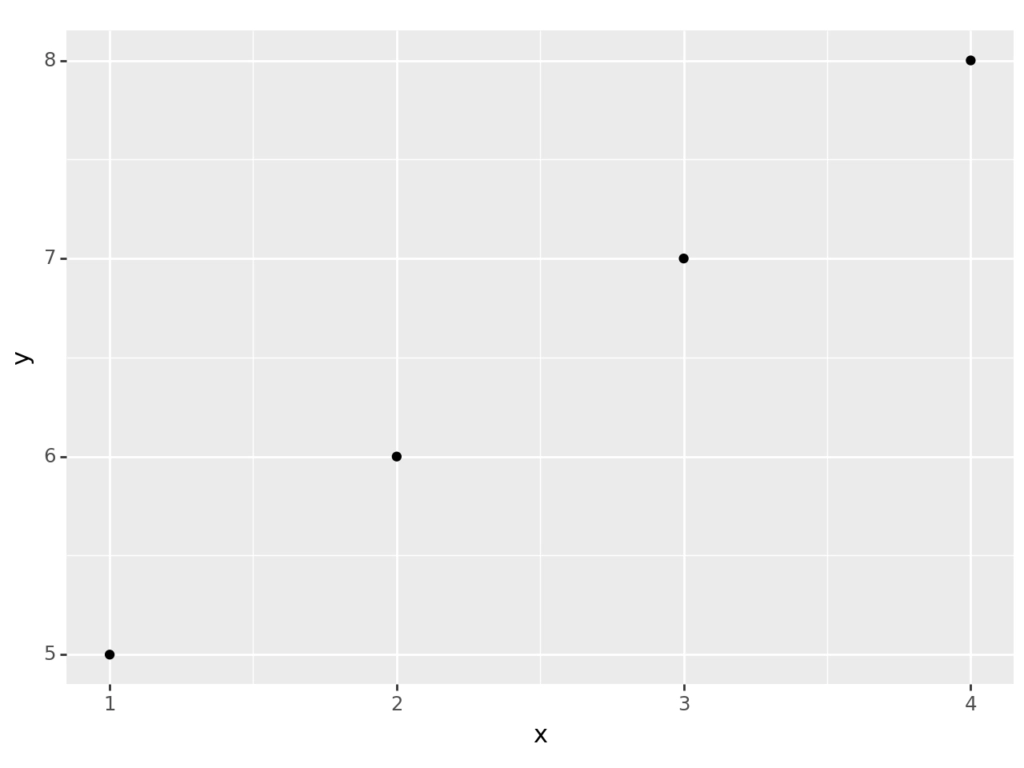
However, if we assign an invalid color to the col argument, such as “NA” or “foo”, we will get the error:
(p9.ggplot(df, p9.aes(x="x", y="y", col="NA"))
+ p9.geom_point())
Error: must request at least one colour from a hue palette.
(p9.ggplot(df, p9.aes(x="x", y="y", col="foo"))
+ p9.geom_point())
Error: must request at least one colour from a hue palette.
Numeric variable
We will also get the error if we assign a numeric variable to the col or fill argument, such as y. This is because ggplot2 expects a factor variable or a valid color name or code for the col or fill argument, not a numeric variable. For example, if we try to color the points by y, we will get the error:
(p9.ggplot(df, p9.aes(x="x", y="y", col="y"))
+ p9.geom_point())
Error: must request at least one colour from a hue palette.
How to resolve the “Error: must request at least one colour from a hue palette.”?
The simplest way to resolve this error is to make sure that the col or fill argument inside the aes function is assigned a factor variable or a valid color name or code. There are four ways to do this:
Convert to factor
We can convert a numeric variable to a factor variable using the pd.Categorical function. For example, if we want to color the points by y, we can convert y to a factor variable using the following code:
df["y"] = pd.Categorical(df["y"])
(p9.ggplot(df, p9.aes(x="x", y="y", col="y"))
+ p9.geom_point())
This code will produce a plot like this:
Use valid color
We can use a valid color name or code to assign to the color or fill argument, such as “red” or “#FF0000”. For example, if we want to color the points by red, we can use the following code:
(p9.ggplot(df, p9.aes(x="x", y="y"))
+ p9.geom_point(color="red"))
This code will produce a plot like this:
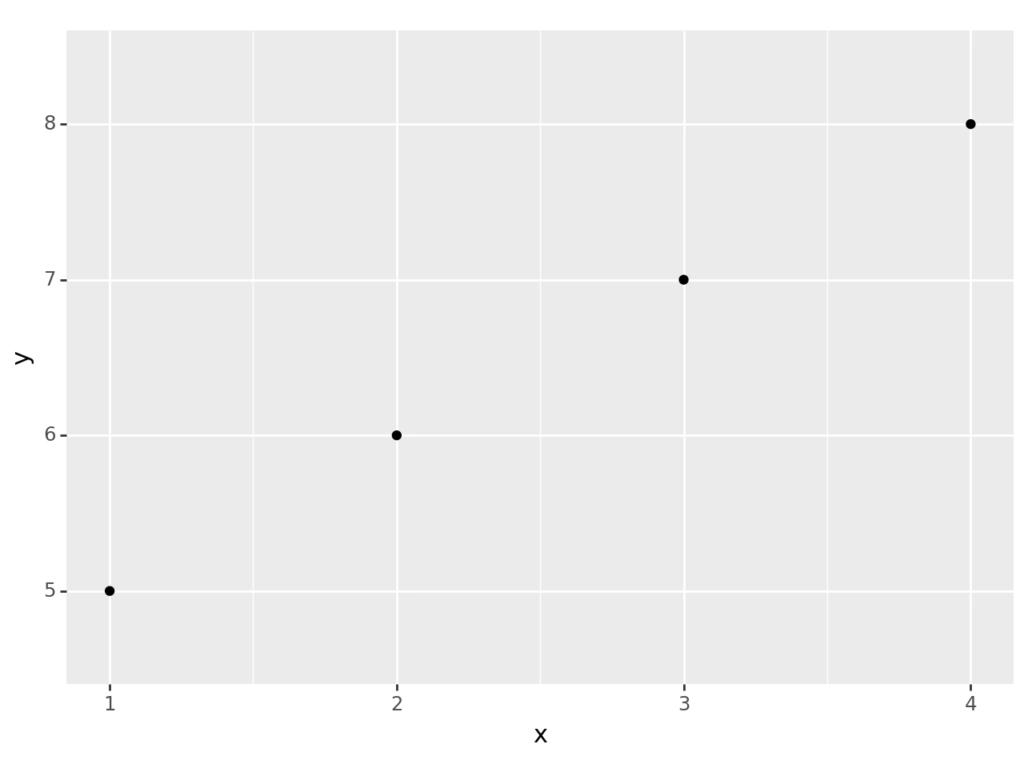
Another way to resolve this error is to use the scale_color_* or scale_fill_* functions to customize the hue palette or the color scale. These functions allow us to change the colors, breaks, labels, limits, and other aspects of color mapping. There are two types of color scales: discrete and continuous. Discrete color scales are used for factor variables, and continuous color scales are used for numeric variables.
Discrete color scale
We can use the scale_color_hue or scale_fill_hue functions to customize the discrete color scale for factor variables. These functions allow us to change the hue range, the chroma, the luminance, the direction, and the breaks of the hue palette. For example, if we want to use a different hue range for the factor variable z, we can use the following code:
(p9.ggplot(df, p9.aes(x="x", y="y", col="z"))
+ p9.geom_point()
+ p9.scale_color_hue(h=360/360))
This code will produce a plot like this:
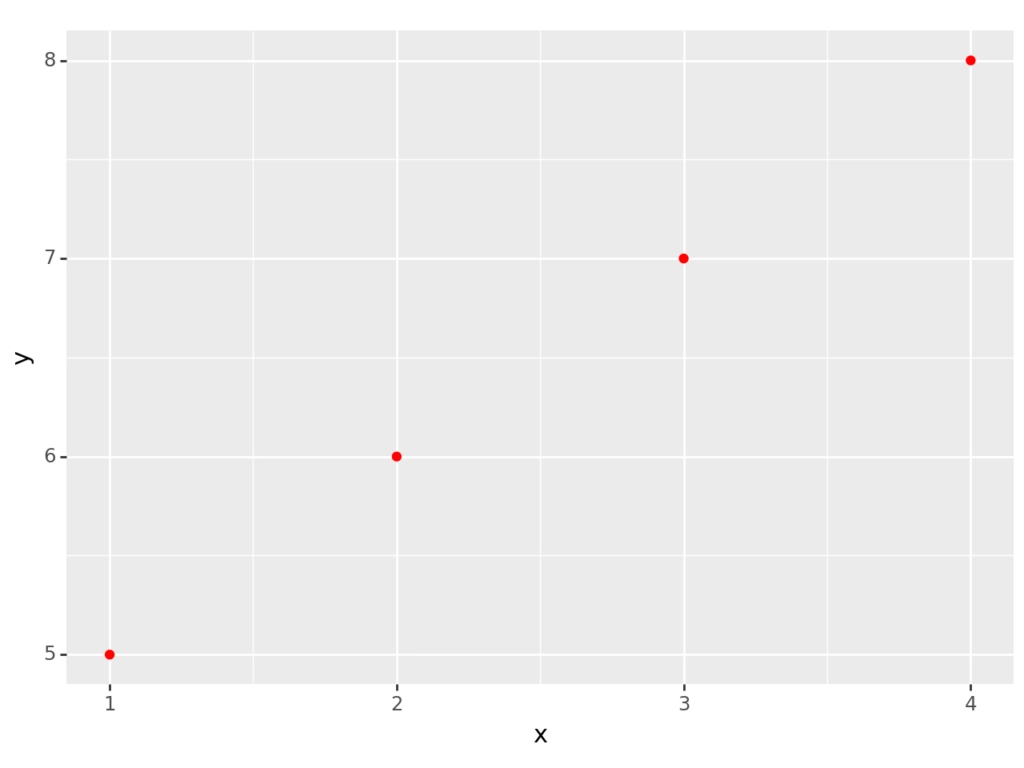
Continuous color scale
We can use the scale_color_continuous or scale_fill_continuous functions to customize the continuous color scale for numeric variables. These functions allow us to change the colors, the breaks, the labels, the limits, and the type of the color scale. For example, if we want to use a gradient color scale for the numeric variable y, we can use the following code:
(p9.ggplot(df, p9.aes(x="x", y="y", color="y"))
+ p9.geom_point()
+ p9.scale_color_gradient(low="blue", high="red"))
This code will produce a plot like this:
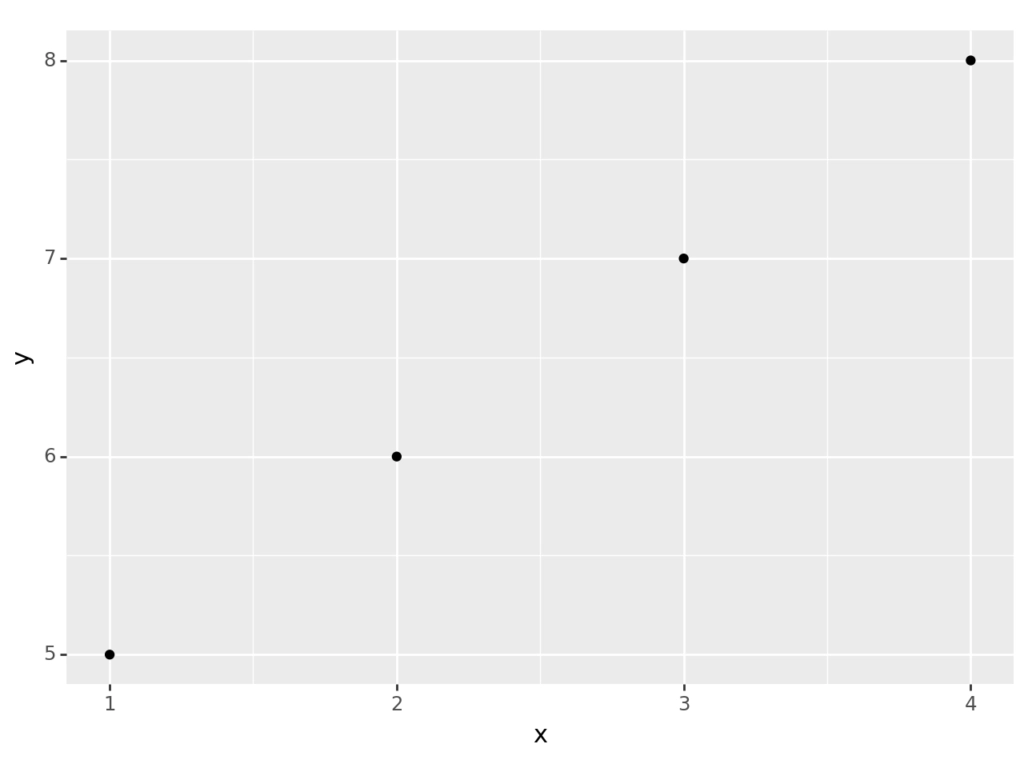
FAQs
What valid color names or codes can I use for the col or fill argument?
You can use any color name or code that is recognized by the matplotlib library, which is the backend for plotnine and ggplot2 in Python. You can use the mentioned codes to find a list of color names and codes.
How can I change the size or shape of the points in the scatter plot?
You can use the size or shape arguments inside or outside the aes function to change the size or shape of the points. For example, you can use p9.aes(size= “x”) to map the size of the points to the x variable or p9.geom_point(size=5) to set the size of the points to 5.
How can I add labels or titles to the plot or the axes?
You can also use the labs function to add labels or titles to the plot or the axes and specify the x, y, title, subtitle, or caption arguments. For example, you can use p9.labs(x=”X axis”, title=”Scatter plot”) to add labels to the x axis and the plot title.
Conclusion
In this article, we have learned what causes the Error: must request at least one colour from a hue palette in Python and how to resolve it using the ggplot2 package. We have also learned how to customize the plots’ color, size, shape, and labels using different functions and arguments. We hope this article has helped you understand and avoid this error in the future and create beautiful and informative plots in Python. Happy plotting!
Reference
Follow us at PythonClear to learn more about solutions to general errors one may encounter while programming in Python.