The Runtimeerror: dictionary changed size during iteration occurs if we tend to change the size of the dictionary while iterating over it. This type of error is an exception error that pops up when the generated error does not fall into any other categories. This error can be resolved by the handlers who are handling exception clauses.
Contents
- 1 What is RuntimeError?
- 2 Causes Of RuntimeError
- 3 What is Runtimeerror: Dictionary Changed Size During Iteration?
- 4 Solution For Runtimeerror: Dictionary Changed Size During Iteration
- 5 FAQ
- 6 How do I get the size of a dictionary in Python?
- 7 How do you check if a key exists in a dictionary in python?
- 8 Conclusion
- 9 References
What is RuntimeError?
The RuntimeError is detected when the raised error does not belong to any other error categories. In this type of error, the values are associated with the strings, which shows the exact cause of the error. This error belongs to the exception error category.
In such errors, even though there are some mistakes in the syntax, it gets a pass until its execution. In this case, the interpreter will find the error and stop it with a message describing the exception.
Causes Of RuntimeError
- This type of error arises if there are some variables and function names that are misspelled or capitalized incorrectly but placed in the correct sequence then the syntax error will not arise, but as soon as you run the program, an error message will pop up, and the program would not be executed.
- Another instance where this type of error would cause is when you try to perform operations on the wrong data type.
- This type of error can also arise when the values which can not be converted into any integer get used with the “int” function.
What is Runtimeerror: Dictionary Changed Size During Iteration?
The runtimeerror: dictionary changed size during iteration is the error that arises in python when we change the size of the dictionary, frequently used data structure during iteration.
If you are someone new and perhaps not acquainted with the terms, then here is a little help for you. Basically, Iteration is a term that is used when the sequences of instructions and codes are put until it gives an iterator as a result. Sometimes people confuse the term iterator with the term iterable due to its common nature. But these two terms have two completely different meanings. The Iterable is an object which uses the “__iter__” command to return an Iterator.
The runtimeerror: dictionary changed size during iteration is a self-explanatory error message which will arise when you try to add any entries during iteration. While you are looping in the iteration, you are not allowed do any kind of changes in the dictionary; hence when you try to do so, it generates the runtimeerror: dictionary changed size during iteration message.
E.g.
If someone wants to delete a value from the dictionary, the runtimeerror: dictionary changed size during iteration error will be caused.
my_dict = { 'x' : 1, 'y' : 2, 'z' : 3, 'a' : 4}
for i in my_dict:
del my_dict["a"]
print (my_dict)
Output:
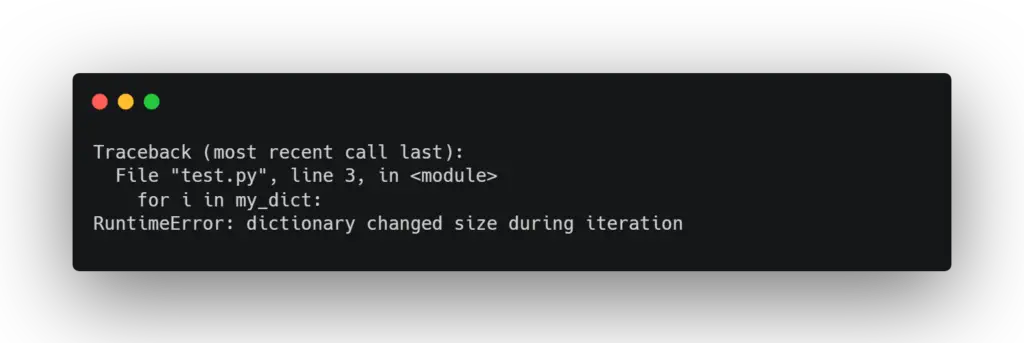
Solution For Runtimeerror: Dictionary Changed Size During Iteration
If you face any runtimeerror: dictionary changed size during iteration error, you can use one of the methods mentioned below.
Making a shallow copy
By making a shallow copy, you can store the references of the original elements in the dictionary. it basically does not create or recurse the nested objects but stores their references. It is not the most convenient method, but it can surely create a solution for the runtimeerror: dictionary changed size during iteration error.
E.g.:
my_dict = { ' x ' : 1, ' y ' : 2, ' z ' : 3, ' a' : 4}
my_dict_copy = my_dict.copy()
for i in my_dict:
del my_dict_copy[i]
print (my_dict)
print(my_dict_copy)
Making a copy of Dictionary Keys
Instead of making a copy of the entire dictionary which can be a bit of a mess, you can create a copy of the dictionary keys and use them as iterators. This can give you a proper solution to the error message without taking up extra space as it will create only a copy of the required keys.
E.g.:
my_dict = { ' x ' : 1, ' y ' : 2, ' z ' : 3, ' a' : 4}
key_copy = tuple(my_dict.keys())
for a in key_copy:
del my_dict[a]
Using Deferred Update
If you want to take other ways than just creating copies of the present dictionary, you can opt for the deferred update method. This method prohibits any changes made during the programming from being applied to the database till the iteration is over. It is the most effective way to avoid the runtimeerror: dictionary changed size during iteration error message.
E.g.:
my_dict = { ' x ' : 1, ' y ' : 2, ' z ' : 3, ' a' : 4}
keys_to_be_removed = []
for i in my_dict:
keys_to_be_removed.append(i)
for a in keys_to_be_removed:
del my_dict[a]
FAQ
How to differentiate RuntimeError from SyntaxError?
A runtimeError is basically the error caused due to misspelling or misplacement in the syntax, which gets a pass for syntaxError but does not run. But on the other hand, the syntaxError is generally related to the placement and use of commands which get caught by the interpreter or compiler.
How do I get the size of a dictionary in Python?
To find the size of the dictionary, you can use the len() function. This function will return the size of the dictionary in terms of the number of key-value pairs present in the dictionary.
E.g.
my_dict = { ' x ' : 1, ' y ' : 2, ' z ' : 3, ' a' : 4}
print("The length of the dictionary is {}".format(len(my_dict)))
How do you check if a key exists in a dictionary in python?
You can check the existence of a key in a python dictionary using the has_key() method. If the key is available, it will return the output as a true or otherwise a false.
E.g.
def checkKey(dic, key):
if dic.has_key(key):
print("Present, value =", dic[key])
else:
print("Not present")
# Driver Function
my_dict = {'x': 1, 'b':2, 'z':3}
key = 'b'
checkKey(dic, key)
key = 'w'
checkKey(dic, key)
Conclusion
This article will help you find the cause for the RuntimeError: dictionary changed size during the iteration and will help you with its solution.
References
Learn more about error handling at PythonClear