The IndexError: Tuple Index Out Of Range error is a common type of index error that arises while dealing with tuple-related codes. These errors are generally related to indexing in tuples which are pretty easy to fix.
This guide will help you understand the error and how to solve it.
Contents
What is a Tuple?
Tuples are one of the four built-in single-variable objects used to store multiple items. Similar to other storage for data collection like List, Sets, and, Dictionary, Tuple is also a storage of data that is ordered and unchangeable. Similar to lists, the items here are also indexed. It is written with round brackets. Unlike other storage data sets, Tuples can have duplicate items, which means the data in a tuple can be present more than once. Also, Tuples can have different data types, such as integers, strings, booleans, etc.
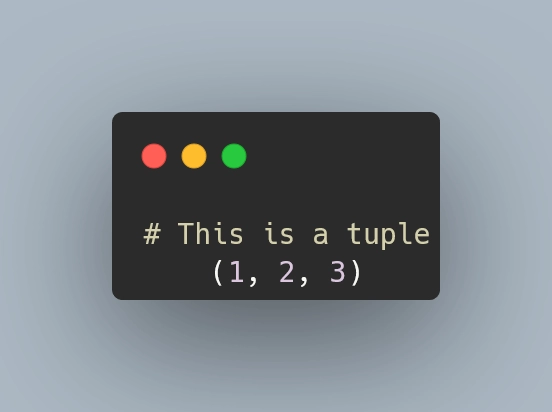
To determine the length of a tuple, the len() function is used. And to get the last item in a tuple, you need to use [- index number of the item you want].
Example: –
a_tuple = (0, [4, 5, 6], (7, 8, 9), 8.0)
What is IndexError?
An IndexError is a pretty simple error that arises when you try to access an invalid index. It usually occurs when the index object is not present, which can be caused because the index being more extensive than it should be or because of some missing information. This usually occurs with indexable objects like strings, tuples, lists, etc. Usually, the indexing starts with 0 instead of 1, so sometimes IndexError happens when one individual tries to go by the 1, 2, 3 numbering other than the 0, 1, 2 indexing.
For example, there is a list with three items. If you think as a usual numbering, it will be numbered 1, 2, and 3. But in indexing, it will start from 0.
Example: –
x = [1, 2, 3, 4]
print(x[5]) #This will throw error
What is IndexError: Tuple Index Out Of Range?
The IndexError: tuple index out-of-range error appears when you try to access an index which is not present in a tuple. Generally, each tuple is associated with an index position from zero “0” to n-1. But when the index asked for is not present in a tuple, it shows the error.
Causes for IndexError: Tuple Index Out Of Range?
In general, the IndexError: Tuple Index Out Of Range error occurs at times when someone tries to access an index which is not currently present in a tuple. That is accessing an index that is not present in the tuple, which can be caused due to the numbering. This can be easily fixed if we correctly put the information.
This can be understood by the example below. Here the numbers mentioned in the range are not associated with any items. Thus this will raise the IndexError: Tuple Index Out Of Range.
Syntax:-
fruits = ("Apple", "Orange", "Banana")
for i in range(1,4):
print(fruits[i]) # Only 1, and 2 are present. Index 3 is not valid.
Solution for IndexError: Tuple Index Out Of Range
The IndexError: tuple index out-of-range error can be solved by following the solution mentioned below.
For example, we consider a tuple with a range of three, meaning it has three items. In a tuple, the items are indexed from 0 to n-1. That means the items would be indexed as 0, 1, and 2.
In this case, if you try to access the last item in the tuple, we need to go for the index number 2, but if we search for the indexed numbered three, it will show the IndexError: Tuple Index Out Of Range error. Because, unlike a list, a tuple starts with 0 instead of 1.
So to avoid the IndexError: Tuple Index Out Of Range error, you either need to put the proper range or add extra items to the tuple, which will show the results for the searched index.
Syntax: –
fruits = ("Apple", "Orange", "Banana")
for i in range(3):
print(fruits[i])
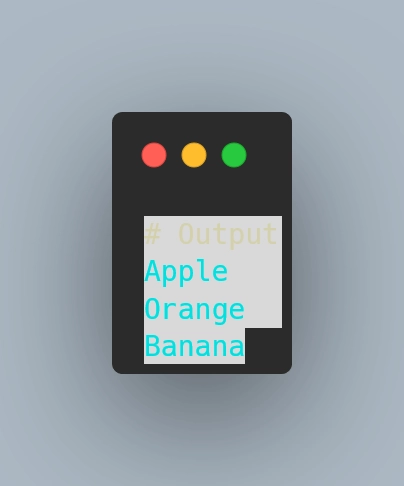
FAQs
What is range()?
The range function in python returns the sequences of numbers in tuples.
What makes tuple different from other storages for data sets?
Unlike the other data set storages, tuples can have duplicate items and use various data types like integers, booleans, strings, etc., together. Also, the tuples start their data set numbering with 0 instead of 1.
How can the list be converted into a tuple?
To convert any lists into a tuple, you must put all the list items into the tuple() function. This will convert the list into a tuple.
Conclusion
The article here will help you understand the error as well as find a solution to it. Apart from that, the IndexError: tuple index out-of-range error is a common error that can be easily solved by revising the range statement or the indexing. The key to solving this error is checking for the items’ indexing.
References
To learn more about some common errors follow Python Clear’s errors section.