ValueError: no JSON object could be decoded error is a type of exception error caused when a file object is called with a JSON.load() or when a string containing the attached JSON code is called with a JSON.loads(). ValueError: no JSON object could be decoded error is an exception error, but it is not included in the IndexError.
In this article, you will understand the ValueError: no JSON object could be decoded error and help you resolve that.
Contents
What is ValueError?
ValueError is a type of exception error in python. It is raised when a function receives an argument of the correct type with an inappropriate value. This type of error should not be put under errors like IndexError.
What is JSON?
JSON in python stands for JavaScript Object Notation, a standardized format commonly used for transferring data as text which can be sent over a network and used by many APIs and databases. These notations are easily understandable for humans as well as machines. It represents objects similar to a python dictionary, i.e., as name or value pairs.
JSON is a text or syntax written by JavaScript object notations and used for storing and exchanging data. It is a built-in package and is used as the JSON module. The JSON module can convert the data into python objects from JSON strings and vice versa.
You may need to use the JSON.loads() operation to convert JSON strings to Python objects.
Syntax:
import json
x = '{ "name":"Jen", "age":60}'
y = json.loads(x)
print(y["name"])
Output:
Jen
You may need to use JSON to convert Python objects to JSON strings.dumps() operation.
Syntax:
import json
x = {"name": "Jen",
"age": 60}
y = json.dumps(x)
print(y)
What is ValueError: no JSON object could be decoded?
r: no JSON object could be decoded in python is a type of ValueError caused when there is an error regarding the conversion of data from the python object to JSON string or vice versa. Sometimes the error causes due to the misplacement or mispronunciation of the conversion operations.
Cause for ValueError: no JSON object could be decoded
The value error: no JSON object could be decoded; it can be caused when a file object is called with a JSON.load() or when a string containing the attached JSON code is called with a JSON.loads(). This type of error will raise an exception.
Syntax:
import json
with open("pc.txt", "r") as f:
p = json.loads(f.read())
print(p)
Output
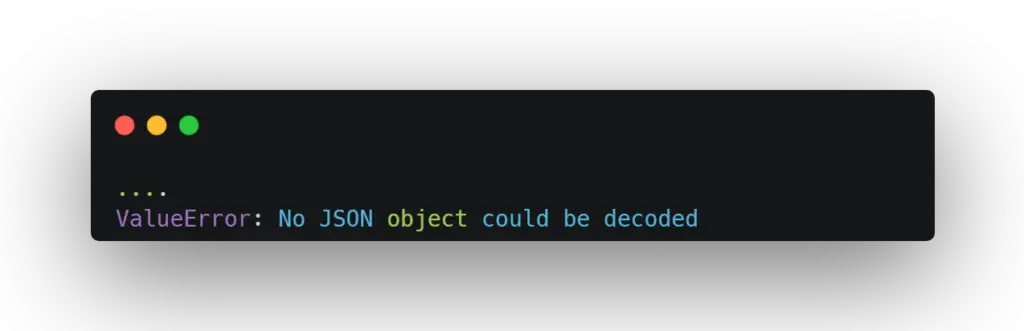
The pc.txt contents should be:
{ "user" : "radist",
"role" : "admin"}
The above code will show the ValuEerror: no JSON object could be decoded if the pc.txt has encodings other than utf-8.
Solution for ValuEerror: no JSON object could be decoded
The above Valueerror: no JSON object could be decoded error can be resolved by following the solution below. These solutions are easy to execute if you know the sources.
Converting JSON file to utf-8 file
The Valueerror: no JSON object could be decoded error caused due to the error in converting JSON strings into python objects can be resolved if the JSON file can be converted into UTF_8 without BOM. It is one of the easiest for the conversion of data and avoiding the value error: no JSON object could be decoded error. For this conversion, you can also use Notepad++.
Syntax:
myFile=open(cases2.json, 'r')
myObject=myFile.read()
u = myObject.decode('utf-8-sig')
myObject = u.encode('utf-8')
myFile.encoding
myFile.close()myData=json.loads(myObject,'utf-8')
Use a valid JSON file.
Sometimes the contents of the JSON file may be errored or invalid due to mispronunciation or lack of correct strings or the presence of any errored template or specification. So checking the JSON file for the validation is a way to avoid any ValuEerror: no JSON object could be decoded error message. You can use the JSON validator to check if the JSON file is valid. For example
FAQs
What is BOM?
The BOM (Byte Order Mark) is a character that can be placed at the beginning of a Unicode text file to show the sequence of the stored bytes.
Is JSON a python library?
JSON is a data format, whereas the python library is a collection of related modules. So no, the JSON is not a python library but similar to the python dictionary.
What is the use of JSON?
JavaScript Object Notation or JSON is a syntax for storing and exchanging data. It represents objects in terms of name or value pairs, similar to the python dictionary. The JSON format can store and transfer the data over the network in text format.
Conclusion
This guide can help you find the causes and the possible solution for the ValueError: no JSON object could be decoded error. The error is also related to the versions of python, so you may check the version you are using along with the other causes.
References
To remain up to date, follow PythonClear/Errors for error resolving.