The “RuntimeWarning: divide by zero encountered in log” warning message typically occurs when there is an attempt to perform a mathematical operation that involves dividing a value by zero and then taking the logarithm of the result. This is not actual error but warning. Since dividing by zero is undefined in mathematics, the logarithm operation cannot be performed, resulting in the warning message.
Contents
- 1 Example showing RuntimeWarning: divide by zero encountered in log
- 2 Situations where this warning occurs
- 3 The occurrence of RuntimeWarning: divide by zero encountered in log
- 4 Approaches to avoid the RuntimeWarning: divide by zero encountered in log
- 5 RuntimeWarning: divide by zero encountered in log logistic regression
- 6 RuntimeWarning: divide by zero encountered in log statsmodels
- 7 RuntimeWarning: divide by zero encountered in log power transformer
- 8 FAQs
- 9 Conclusion
- 10 References
Example showing RuntimeWarning: divide by zero encountered in log
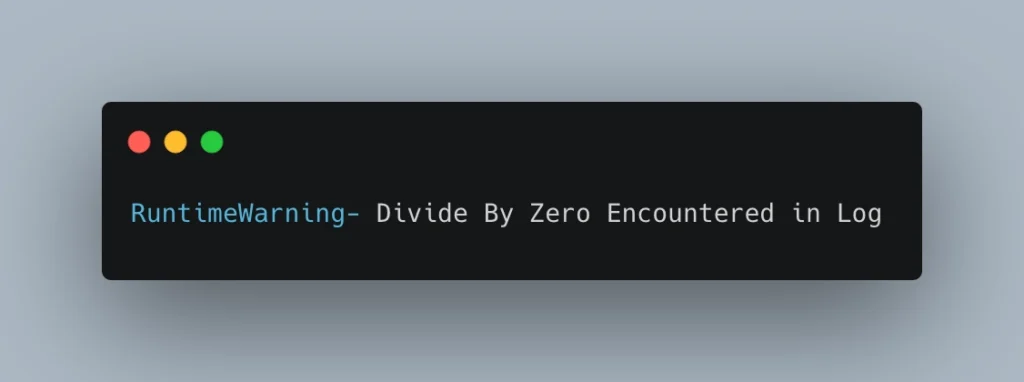
The below example displays the error ‘RuntimeWarning: divide by zero encountered in log’ once the code is executed.
Syntax:
import numpy as np
x = 0
result = np.log(x)
Situations where this warning occurs
The “RuntimeWarning: divide by zero encountered in log” warning can occur in various scenarios where a logarithmic operation involves dividing a value by zero. Here are a few common scenarios that can trigger this warning:
- Division by zero: When explicitly dividing a number by zero, such as
x/0
, the result is undefined, and attempting to compute the logarithm of the result will raise the warning. - Mathematical calculations: In mathematical calculations or formulas that involve division, if a denominator evaluates to zero, it can lead to the divide-by-zero scenario when applying a logarithm operation.
- Data-dependent calculations: If you have a calculation that involves variables or data inputs, and some of those inputs can potentially be zero, it’s important to handle the scenario where the denominator becomes zero to avoid the divide-by-zero warning.
- Numerical instability or edge cases: In certain mathematical functions or algorithms, there can be edge cases or numerical instabilities that result in division by zero situations when computing logarithms, leading to the warning.
The occurrence of RuntimeWarning: divide by zero encountered in log
The prevalence of the “RuntimeWarning: divide by zero encountered in log” warning depends on the specific code and data being processed. The frequency of encountering this warning can vary based on factors such as the input data, the logic of the code, and the specific computations being performed. If the code involves calculations that can result in division by zero, and if one is applying the logarithm operation on the result, we may encounter this warning more frequently.
The warning is triggered whenever the code encounters a situation where the logarithm operation is attempted on a zero or zero-derived value. It’s important to handle or avoid division by zero scenarios to prevent this warning from occurring.
Approaches to avoid the RuntimeWarning: divide by zero encountered in log
Check for zero before computing the logarithm: Before performing the logarithm operation, you can include a conditional statement to verify if the value is zero and handle it appropriately. By doing so, you can prevent the division by zero error and its corresponding warning message.
Syntax:
import numpy as np
x = 0
if x != 0:
result = np.log(x)
else:
result = 0 # or any other suitable value
Substitute zero with a small positive value: Instead of explicitly checking for zero and handling it separately, you can replace zero values with a small positive value to avoid division by zero altogether. By doing this, you ensure that the logarithm operation can be performed without encountering the warning. By replacing zero with 1e-10
, which represents a very small positive value, we can avoid the divide-by-zero situation.
import numpy as np
x = 0
x = np.where(x == 0, 1e-10, x) # Replace zero values with a small positive value
result = np.log(x)
RuntimeWarning: divide by zero encountered in log logistic regression
In logistic regression, we often encounter the RuntimeWarning: divide by zero encountered in log
warning, which occurs when we try to calculate the logarithm of zero or a negative value. Since logistic regression involves calculating the logarithm of odds, this warning arises when the odds approach zero.
It is important to keep in mind that addressing this warning requires a case-specific approach, and the most suitable solution depends on the characteristics of our data and the goals of our analysis.
RuntimeWarning: divide by zero encountered in log statsmodels
In the context of statsmodels, the RuntimeWarning: divide by zero encountered in log
warning typically arises when utilizing a logistic regression model to fit data containing zero or negative values in the dependent variable.
It’s crucial to recognize that this warning in logistic regression is highly dependent on the specific characteristics of your data and the objectives of your analysis.
RuntimeWarning: divide by zero encountered in log power transformer
The RuntimeWarning: divide by zero encountered in log
warning in the context of a power transformer typically occurs when attempting to compute the logarithm of zero or a negative value. This warning is likely triggered when applying a power transformation to your data that involves logarithmic operations.
It is important to note that addressing the RuntimeWarning: divide by zero encountered in log
warning in power transformation is case-specific and depends on the nature of your data. Consider the characteristics of your dataset and the objectives of your analysis when choosing the appropriate strategy for handling zero or negative values.
FAQs
What are Runtime warnings?
Runtime warnings are meant to draw attention to certain situations that might need further investigation or handling. They can be related to various aspects of program execution, such as mathematical operations, type conversions, deprecated functionality, or other runtime behaviors.
Why Dividing by zero is not accepted in Python?
Dividing a number by zero is an operation that is mathematically undefined. It violates the fundamental principles of arithmetic and leads to an error or an undefined result. In most programming languages, attempting to divide a number by zero will result in an error or an exception
Conclusion
Overall, to address this warning, we can employ techniques such as checking for zero values, using conditional statements to handle specific cases, substituting zero with a small positive value, or redesigning the calculation to avoid the divide-by-zero scenario altogether.
The approach to take depends on the specific context and requirements of the code. To minimize the frequency of encountering this warning, we can incorporate appropriate checks and error handling mechanisms in your code to handle zero values before applying the logarithm operation.
References
- Python Docs – RuntimeWarning
More Python Errors here.