“typeerror: ‘builtin_function_or_method’ object is not subscriptable” to understand this error, first, we need to learn what are subcriptable objects in Python, how to subscript (or how to index, to be more precise), what does interable and subscriptable mean.
Contents
What are subscriptable objects in python?
To learn what “typeerror: ‘builtin_function_or_method’ object is not subscriptable” is we need to understand what are subsciptable objects in python. Subsciptable objects typically contain single or multiple data entries such as strings, lists, tuples, arrays, etc. You can access the entries in the object by using []
(square brackets). In general, objects that do not contain any form of arrangement of data are not subscriptable for example functions.
Difference between Subscriptable and Iterable objects.
A subscriptable object can be indexed by a sequence of integers. The indexing notation obj[seq]
requests the value of the object at the position given by seq
. If the index is out of bounds, an IndexError
exception is raised.
An Iterable is any object in Python that can be looped over. This means that an iterable can be passed to a function like map or filter and you will get an iterator back. Additionally, any object with a __iter__
method can be passed to the built-in function iter and you will get an iterator.
Some objects can be subscriptable and iterable, while others are neither.
When does “typeerror: ‘builtin_function_or_method’ object is not subscriptable” occur?
This error occurs when you try to use square brackets ([]
), curly braces ({}
), or parentheses ()
on a built-in function or method. For example, if you try to do the following:
print[len([1, 2, 3])]
You will get the following error:
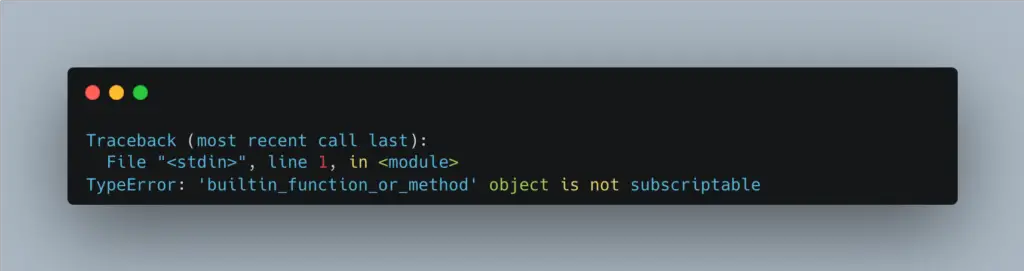
If you're not familiar with the term "subscriptable", it just means that you cannot use square brackets ([]
), curly braces ({}
), or parentheses()
on an object.
So, for example, the following would be valid in Python:
my_list = [1, 2, 3]
print(my_list[0])
But the following would give you the same error:
my_list = [1, 2, 3]
print(my_list[0][0])
Similarly, the following would be valid Python:
my_dict = {"a": 1, "b": 2, "c": 3}
print(my_dict["a"])
But the following would give you an error:
my_dict = {"a": 1, "b": 2, "c": 3}
print(my_dict["a"]["b"])
So, when you try to use square brackets ([]
), curly braces ({}
), or parentheses ()
On a built-in function or method, you get the “typeerror: ‘builtin_function_or_method’ object is not subscriptable” error.
How to fix the typeerror: ‘builtin_function_or_method’?
Fortunately, this error is easy to fix. If you want to use square brackets ([]
), curly braces ({}
), or parentheses ()
on a built-in function or method, you need to wrap the function or method in a Python object.
For example, if you want to use the len()
function with square brackets ([]
), you can do the following:
my_list = [1, 2, 3]
print(len(my_list))
If you want to use the len()
function with curly braces ({}
), you can do the following:
my_dict = {"a": 1, "b": 2, "c": 3}
print(len(my_dict))
If you want to use the len()
function with parentheses ()
, you can do the following:
my_tuple = (1, 2, 3)
print(len(my_tuple))
As you can see, all you need to do is wrap the built-in function or method in the appropriate Python object (list, dictionary, tuple, etc.) and then you can use square brackets ([]
), curly braces ({}
), or parentheses ()
on the function or method.
Conclusion
In this article, we have explained what subsciptable objects are in Python, the difference between subsciptable and iterable objects, what causes the “typeerror: ‘builtin_function_or_method’ object is not subscriptable” error, and how to fix it.
We hope you now understand this error better and how to avoid it in the future.
References
Here’s a post on how to prepend lists in python. Click on the link to learn more.
That’s it for this post. Keep reading Python Clear to gain more Python Knowledge!