The mysterious “TypeError: ‘numpy.float64’ object is not callable” can perplex Python developers, especially those exploring the intricacies of numerical computing with NumPy. In this in-depth guide, we will not only dissect the roots of this error but also scrutinize its various causes, providing practical and nuanced solutions. By delving into advanced techniques and additional considerations, this comprehensive guide aims to equip developers with a robust toolkit to navigate and resolve this coding challenge gracefully.
Contents
- 1 Understanding Python’s “TypeError: ‘numpy.float64’ object is not callable”
- 2 Causes of Python’s”TypeError: ‘numpy.float64’ object is not callable”
- 3 Resolving the “TypeError: ‘numpy.float64’ object is not callable”
- 3.1 Avoiding Overriding Built-in Functions
- 3.2 Ensuring the ‘self’ Argument in Class Methods
- 3.3 Using a Static Method
- 3.4 Specifying the Third Argument in Function Definitions
- 3.5 Avoid Passing Extra Arguments to Functions
- 3.6 Leveraging NumPy Functions Appropriately
- 3.7 Verifying NumPy Version Compatibility
- 3.8 Optimizing NumPy Function Calls
- 4 FAQs
- 5 Conclusion:
- 6 Reference
Understanding Python’s “TypeError: ‘numpy.float64’ object is not callable”
The enigmatic “TypeError: ‘numpy.float64’ object is not callable” surfaces when a NumPy float64 object, not intended to be callable like a function, is mistakenly invoked as such in the code. This error, categorized under TypeErrors in Python, necessitates a nuanced understanding for effective resolution.NumPy’s float64 objects are data types representing double-precision floating-point numbers. They serve as containers for numerical data and support various mathematical operations. However, attempting to call a NumPy float64 object as if it were a function goes against its nature and triggers this specific error.
To delve deeper, grasping the concept of “callable” objects in Python is essential. Callable objects can be invoked using parentheses, such as functions or certain class instances. NumPy float64 objects do not fall into this category; they are not designed to execute code when called. This error often arises when developers mistakenly treat NumPy arrays or elements as callable functions. It can manifest in various scenarios, from attempting to invoke an entire array to poorly considering a specific element as a callable function.
Causes of Python’s”TypeError: ‘numpy.float64’ object is not callable”
Overriding a NumPy Function
A typical scenario leading to this error is attempting to override a built-in NumPy function, inadvertently treating it as a callable object in the code.
Syntax:-
import numpy as np
class MathOperations:
def add_numbers(a, b):
return a + b
np.float64.__call__ = None
result = MathOperations.add_numbers(3, 4)
Missing ‘self’ Argument in a Class Method:
Omitting the ‘self‘ argument in a class method, mainly when dealing with NumPy objects, can be a key trigger for this error.
Syntax:-
import numpy as np
class MathOperations:
def add_numbers(a, b):
return a + b
# Create an instance of the MathOperations class
math_operations = MathOperations()
# Call the add_numbers method using the instance
result = math_operations.add_numbers(3, 4)
Resolving the “TypeError: ‘numpy.float64’ object is not callable”
You can use one of the many solutions mentioned below to Resolve the Python “TypeError: ‘numpy.float64’ object is not callable”.
Avoiding Overriding Built-in Functions
Overriding a built-in NumPy function is discouraged. Ensuring your code doesn’t inadvertently redefine or override NumPy functions is a preventive measure.
Ensuring the ‘self’ Argument in Class Methods
When defining class methods involving NumPy objects, ensure the ‘self’ argument is included to preemptively address the error.
Syntax:-
import numpy as np
class MathOperations:
def add_numbers(self, a, b):
return a + b
math_instance = MathOperations()
result = math_instance.add_numbers(3, 4)
print(result)
Using a Static Method
For scenarios where the ‘self’ argument is not preferred, declaring a static method allows the method to be called on the class or an instance without the ‘self’ argument.
Syntax:-
import numpy as np
class MathOperations:
def add_numbers(a, b):
return a + b
result = MathOperations.add_numbers(3, 4)
print(result)
Specifying the Third Argument in Function Definitions
If a function is expected to take three arguments, ensure all three are provided during the function call to mitigate the error.
Syntax:-
import numpy as np
def perform_calculation(a, b, c):
return a + b + c
result = perform_calculation(3, 4, 5)
print(result)
Avoid Passing Extra Arguments to Functions
Exercise caution not to pass more arguments than a function expects. NumPy functions, in particular, may only accept a specific number of arguments.
To fully understand and address the “TypeError: ‘numpy.float64’ object is not callable,” exploring additional strategies is essential. Let’s delve into some advanced techniques to enhance your problem-solving toolkit.
Leveraging NumPy Functions Appropriately
Ensure a clear understanding of how NumPy functions operate and avoid unnecessary attempts to override or modify their behavior. Utilize NumPy functions as intended, enhancing code readability and maintainability.
Verifying NumPy Version Compatibility
Sometimes, version mismatches between NumPy and other dependencies can contribute to unexpected errors. Regularly check for updates and ensure compatibility between your code and the NumPy version.
Optimizing NumPy Function Calls
Optimize your usage of NumPy functions by reviewing the documentation and exploring alternative functions that may better suit your requirements. NumPy provides a rich set of functions, and choosing the right one can enhance performance and code clarity.
Using the np.min function
One such example of the NumPy Function Calls is the np.min Function, which can resolve this error. The np.min function is usually used to return the minimum of an array.
Syntax:
import numpy as np
def perform_calculation(a, b, c):
return (a + b + c) / 3
a = 3
b = 4
c = 5
result_min = np.min([a, b, c])
print("Minimum:", result_min)
The above code will return the minimum value without raising the TypeError: ‘numpy.float64’ object is not callable.
FAQs
What is a positional argument?
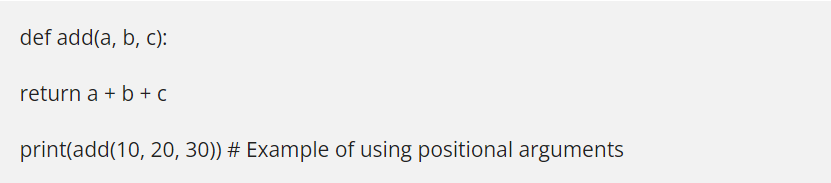
A positional argument is passed during a function call, which must align with the order of parameters in the function definition.
Syntax:
What is the ‘self’ in Python?
‘Self’ in Python refers to the class instance. It is used to access attributes and methods of the class and to bind attributes with a given argument.
Conclusion:
In conclusion, the journey to resolve the “TypeError: ‘numpy.float64’ object is not callable” is multifaceted. By understanding its origins, implementing best practices, and exploring advanced strategies, Python developers can fortify their ability to tackle this specific error. Navigating the complexities of numerical computing with NumPy becomes more manageable, contributing to a more robust and efficient Python programming experience. As the Python ecosystem evolves, continuous exploration and adaptation are key to mastering such challenges and becoming proficient.
Reference
Follow us at PythonClear to learn more about solutions to general errors one may encounter while programming in Python.