One of the common errors that you may encounter when working with pandas DataFrames in Python is the ValueError: cannot set a row with mismatched columns. This error often occurs when you try to add a new row to an existing DataFrame, but the number of values in the new row does not match the number of columns in the DataFrame. This article will give you a walkthrough about the causes of this error, how to avoid it, and how to fix it if it happens.
Contents
- 1 What is the ValueError: cannot set a row with mismatched columns error?
- 2 What causes the ValueError: cannot set a row with mismatched columns error?
- 3 How to avoid the ValueError: cannot set a row with mismatched columns error?
- 4 How to fix the ValueError: cannot set a row with mismatched columns error?
- 5 FAQs
- 6 Conclusion
- 7 Reference
What is the ValueError: cannot set a row with mismatched columns error?
The ValueError: cannot set a row with mismatched columns error is a type of ValueError that is raised by pandas when you try to assign a row to a DataFrame using the loc or iloc methods, but the length of the row is different from the number of columns in the DataFrame. For example, suppose we have the following DataFrame:
import pandas as pd
# create DataFrame
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'David', 'Eve'],
'age': [25, 30, 35, 40, 45],
'gender': ['F', 'M', 'M', 'M', 'F']
})
# view DataFrame
df
name | age | gender | |
---|---|---|---|
0 | Alice | 25 | F |
1 | Bob | 30 | M |
2 | Charlie | 35 | M |
3 | David | 40 | M |
4 | Eve | 45 | F |
Now, if we attempt to add a new row to the end of the DataFrame using the loc method, but we only provide two values instead of three, we will get the error:
# define new row to append
new_row = ['Frank', 50]
# append row to DataFrame
df.loc[len(df)] = new_row
# view updated DataFrame
df

The same error will occur if we use the iloc method instead of the loc method, or if we provide more than three values in the new row.
What causes the ValueError: cannot set a row with mismatched columns error?
The ValueError: cannot set a row with mismatched columns error is caused by the fact that pandas expects the row that you assign to a DataFrame to have the same length as the number of columns in the DataFrame. This is because pandas tries to align the values in the row with the columns in the DataFrame by their positions. If the row has fewer values than the columns, pandas does not know how to fill in the missing values. If the row has more values than the columns, pandas does not know how to handle the extra values.
How to avoid the ValueError: cannot set a row with mismatched columns error?
The best way to avoid the ValueError: cannot set a row with mismatched columns error is to make sure that the row that you want to add to a DataFrame has the same length as the number of columns in the DataFrame. You can check the length of the row and the number of columns using the len function:
# check the length of the row
len(new_row)
2
# check the number of columns in the DataFrame
len(df.columns)
3
If the lengths are not equal, you can either modify the row or the DataFrame to make them match. For example, you can add or remove values from the row, or you can add or drop columns from the DataFrame.
How to fix the ValueError: cannot set a row with mismatched columns error?
If you encounter the ValueError: cannot set a row with mismatched columns error, there are a few ways to fix it. One way is to use the append method instead of the loc or iloc methods to add a new row to a DataFrame. The append method will automatically fill in the missing values with NaN, or ignore the extra values, depending on the case. For example, using the same DataFrame and new row as before, we can use the append method as follows:
# define new row to append
new_row = ['Frank', 50]
# append row to DataFrame
df = df.append(pd.Series(new_row, index=df.columns[:len(new_row)]), ignore_index=True)
# view updated DataFrame
df
name | age | gender | |
---|---|---|---|
0 | Alice | 25 | F |
1 | Bob | 30 | M |
2 | Charlie | 35 | M |
3 | David | 40 | M |
4 | Eve | 45 | F |
5 | Frank | 50 | NaN |
Notice that the new row has been appended to the end of the DataFrame, and the missing value in the gender column has been filled with NaN. Alternatively, you can also specify the columns that you want to append the new row to using a dictionary:
# define new row to append
new_row = {'name': 'Frank', 'age': 50}
# append row to DataFrame
df = df.append(new_row, ignore_index=True)
# view updated DataFrame
df
name | age | gender | |
---|---|---|---|
0 | Alice | 25 | F |
1 | Bob | 30 | M |
2 | Charlie | 35 | M |
3 | David | 40 | M |
4 | Eve | 45 | F |
5 | Frank | 50 | NaN |
Another way to fix the error is to use the concat method to concatenate two DataFrames along the row axis. The concat method will also fill in the missing values with NaN, or ignore the extra values, depending on the case. For example, using the same DataFrame and new row as before, we can use the concat method as follows:
# define new row to append
new_row = ['Frank', 50]
# create a new DataFrame from the new row
new_df = pd.DataFrame([new_row], columns=df.columns[:len(new_row)])
# concatenate the two DataFrames
df = pd.concat([df, new_df], ignore_index=True)
# view updated DataFrame
df
name | age | gender | |
---|---|---|---|
0 | Alice | 25 | F |
1 | Bob | 30 | M |
2 | Charlie | 35 | M |
3 | David | 40 | M |
4 | Eve | 45 | F |
5 | Frank | 50 | NaN |
FAQs
How can I fill in the missing values with a specific value instead of NaN?
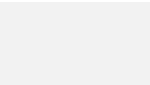
You can use the fillna method to replace the NaN values with a specific value. For example, if you want to fill in the missing values in the gender column with ‘U’ for unknown, you can do:
How can I drop the rows or columns that have missing values?
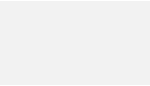
You can use the dropna method to drop the rows or columns that have missing values. For example, if you want to drop the rows that have missing values, you can do:
Conclusion
In this article, we have learned what the ValueError: cannot set a row with mismatched columns error is, what causes it, how to avoid it, and how to fix it in Python. We have seen that this error occurs when we try to add a new row to a pandas DataFrame, where the number of values in the row does not match the number of columns in the DataFrame. We have also learned how to use the append, concat, fillna, and dropna methods to deal with this error. We hope that this article has helped you understand and solve this error in your Python projects.
Reference
Follow us at PythonClear to learn more about solutions to general errors one may encounter while programming in Python.