Have you ever written a Python program that ran into an error and displayed a traceback? If so, you may have seen the error “else do nothing python”. This is a common error that occurs when trying to use an “else
” statement without a corresponding “if
” statement.
Contents
Why does the else do nothing python error occur?
The else do nothing Python error occurs when a programmer uses the “else
” keyword without an accompanying “if
” keyword. This can happen in two ways: either the “else
” is not indented correctly, or it is misplaced.
Incorrect indentation is the most common cause of this error. When an “else
” block is not indented correctly, it is treated as part of the code that precedes it. This can be seen in the following code example
for i in range(5):
print(i)
else:
print("done!")
In this example, the code inside the “else
” block will run every time because it is not part of the for
loop. The correct indentation would be:
for i in range(5):
print(i)
else: # this else is now properly indented
print("done!")
The second way that this error can occur is if the “else
” keyword is misplaced. This can happen if the programmer meant to use a different keyword, such as “elif
“.
For example, consider the following code:
for i in range(5):
print(i)
elif i == 3: # this should be an "else if", not an "elif"!
print("three!")
In this code, the “elif
” is treated as an “else
“, because that is what it comes after. As a result, the code inside the “elif
” block will never run. The correct code would be:
for i in range(5):
print(i)
else:
if i == 3:
print("three!")
How to fix the error?
The most common way to fix this error is to simply check the indentation of your code. Make sure that all of your “else
” blocks are properly indented, and they are not part of any other code blocks.
If you are unsure whether or not you have correctly indented your code, you can always run it through a Python linter. A linter is a program that will check your code for errors and potential problems.
The other way to fix this error is to check your use of keywords. Make sure that you are using the correct keyword for what you want to do. If you are unsure, you can always consult the Python documentation.
More Information
Most developers are familiar with the if
statement in Python. It allows you to check a condition and execute code based on the result. For example:
if (condition):
# do something
elif (condition):
# do something else
else:
# do something else
Often, you may want to exit or continue a for
or while
loop based on a condition. One way to do this is with the continue
keyword. For example:
for i in range(10):
if (i == 5):
continue
print(i)
Gets us the output as follows:
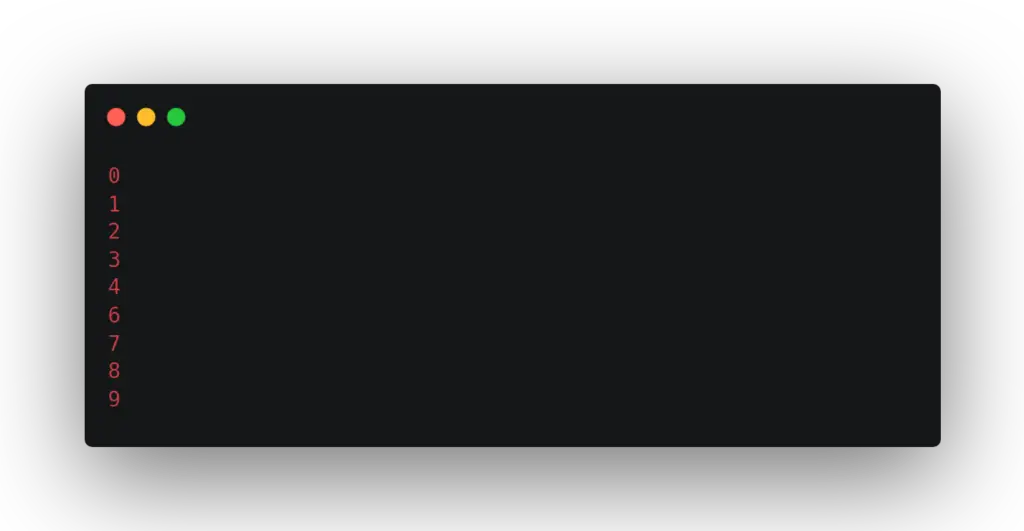
The continue
keyword can be used in both while
and for
loops. It causes the current iteration of the loop to stop and continue with the next iteration.
For example, let’s say we have a list of numbers and we want to print out all the even numbers in the list:
numbers = [1, 2, 3, 4, 5]
for num in numbers:
if num % 2 == 0:
print(num)
else: # this
continue
else
statement will never run because all Even Numbers are printed before this line due to continue
statement on line 5. The above code will give us an output as follows:
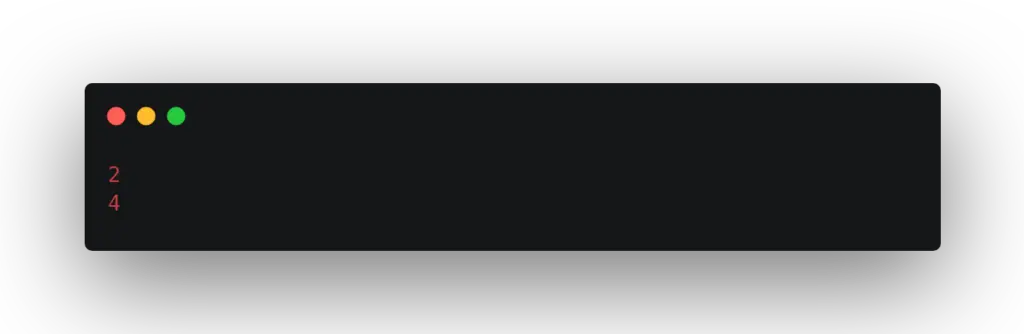
Conclusion
In conclusion, the “else do nothing python” error in Python occurs when a programmer uses the “else
” keyword without an accompanying “if
” keyword. This can happen due to incorrect indentation, or because the programmer meant to use a different keyword such as “elif
“.
The most common way to fix this error is to simply check the indentation of your code. Another way to fix this error is to check your use of keywords and make sure that you are using the correct one for what you want to do.
References
Also see how to fix common errors like can’t find __main__ module, unittest assertraises on pythonclear.