Python can't find '__main__' module
is one of the most common errors that can occur when running a Python script. We’ll cover why this error occurs and provide two different code examples that demonstrate how it can happen. We’ll also show you how to fix the error so that you can continue developing your web applications with Python.
Contents
What is __main__
in Python?
__main__
is a special name in Python that is used to indicate the source file where the program execution starts. Every Python program has a '__main__'
module, and the code within that module is executed when the program is run. The '__main__'
module can be located in any file, but it is typically placed in a file named ‘main.py
‘.
For example, consider the following 'main.py'
file:
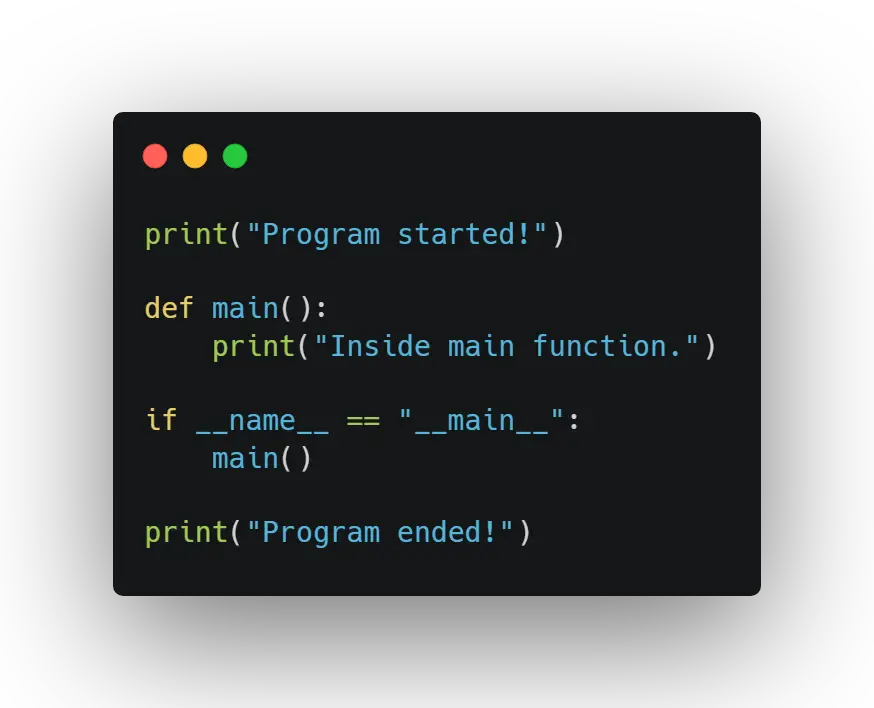
When this program is run, the output will be as follows:
Program started!
Inside main function.
Program ended!
Why does the error occur?
There are two main reasons why the python can't find '__main__' module
error might occur. The first reason is that you haven’t specified the main module in your Python script. The second reason is that your main module is not located in the same directory as your Python script. We’ll discuss each of these reasons in more detail below.
Code example 1
Let’s say you have a Python script named my_script.py
and it contains the following code:
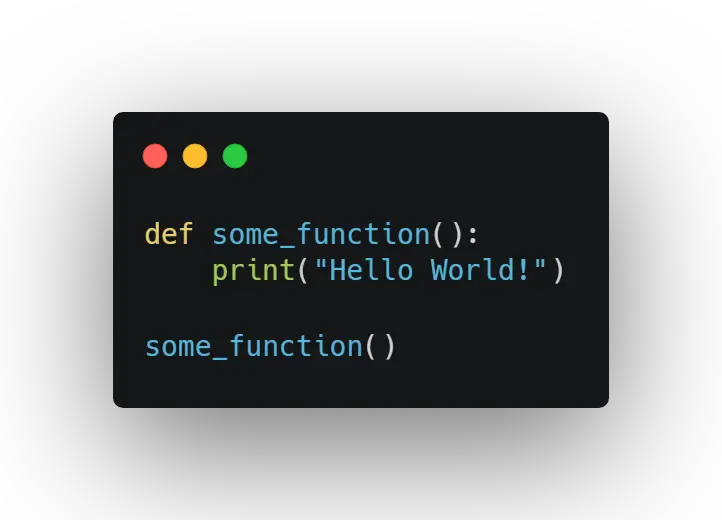
If you try to run this script, you’ll get the python can’t find ‘__main__’ module error
$ python my_script.py
Traceback (most recent call last):
File "my_script.py", line 7, in <module>
some_function()
File "my_script.py", line 4, in some_function
print("Hello World!")
NameError: name '__main__' is not defined
This is because we haven’t specified the main module in our script. Python will look for a module named __main__ and, if it can’t find one, it will throw an error. To fix this, we need to add the main module to our script. We can do this by wrapping our code in an if __name__ == "__main__":
block:
if __name__ == "__main__":
def some_function():
print("Hello World!")
some_function()
Now when we run our script, we get the expected output:
$ python my_script.py
Hello World!
Code example 2
Let’s say you have a Python script named my_script.py
and it contains the following code:
import some_module
def some_function():
print("Hello World!")
some_function()
And you also have a Python module named some_module.py
that contains the following code:
def some_other_function():
print("Hello from some other function!")
If you try to run my_script.py
, you’ll get the following error:
$ python my_script.py
Traceback (most recent call last):
File "my_script.py", line 7, in <module>
import some_module
ImportError: No module named '__main__' in some_module
This is because your main module (my_script.py
) is not located in the same directory as your Python script. When Python tries to import some_module
, it will look for it in the current directory (the directory where my_script.py
is located). Since it can’t find it there, it will throw an error. You can fix this by moving your Python script to the same directory as your main module:
my_script.py
some_module.py
Now when you run my_script.py
, it will be able to find and import some_module.py
without any problems.
How to fix the python can’t find ‘__main__’ module error?
As we’ve seen in the examples above, there are two ways to fix the “python can’t find ‘__main__’ module” error. The first way is to add the main module to your Python script. The second way is to move your Python script to the same directory as your main module. Below, we’ll go through both of these strategies in further depth.
Add the main module to your script
If you get this error, it means that you haven’t specified the main module in your Python script. You can fix this by wrapping your code in an if __name__ == "__main__":
block:
if __name__ == "__main__":
def some_function():
print("Hello World!")
some_function()
When you run your script, Python will look for a module named __main__
and, if it can’t find one, it will throw an error. This block of code will ensure that Python can find your main module and avoid errors like python can't find '__main__' module
Move your script to the same directory as your main module
If you get this error, it means that your main module is not located in the same directory as your Python script. You can fix this by moving your Python script to the same directory as your main module:
my_script.py
some_module.py
When you run your Python script, it will look for modules in the current directory (the directory where my_script.py
is located). By moving my_script.py
to the same directory we’re ensuring that our script can find all of the modules it needs.
Conclusion
In this article, we’ve discussed the “python can't find '__main__' module
” error. We’ve seen two different code examples that demonstrate how this error can occur. We’ve also shown you how to fix the error by either adding the main module to your script or moving your script to the same directory as your main module so that you can continue developing your web applications.
References
To learn more, also follow unittest assertraises(), prepend lists on pythonclear