Python invalid index to scalar variable is an error that might be encountered because various reasons, which we will explore in this article. First, we will discuss this error, its meaning, and why it might occur. Then, we will show some ways to fix this error. Finally, we will offer more information on the topic for those who want to learn more.
Contents
What Does Python Invalid Index to Scalar Variable Mean?
Python invalid index to scalar variable error means that you are trying to use an index (subscript) on a variable that is not an interable object (for example, an array or a list). Check out subscriptable objects to learn more about interable and subscriptable objects. Consider the following example code:
my_list = [1, 2, 3]
print(my_list[0]) # This is valid
my_scalar = 5
print(my_scalar[0]) # This gives an error because my_scalar is not a list
In the first line of code above, we create a list called my_list
. We can then use the square brackets ([ ]
) to get the first element of this list (which has an index of 0). However, if we try to do the same thing with a scalar variable (a single value), it will not work because my_scalar
is not an interable object is an integer. This is an example of what causes the “invalid index to scalar variable” error.
Why Does Python Invalid Index to Scalar Variable Occur?
This error might occur in your code for a few different reasons. For example, you might accidentally try to use an index on a scalar variable:
my_scalar = 5
print(my_scalar[0])
This will give an error:
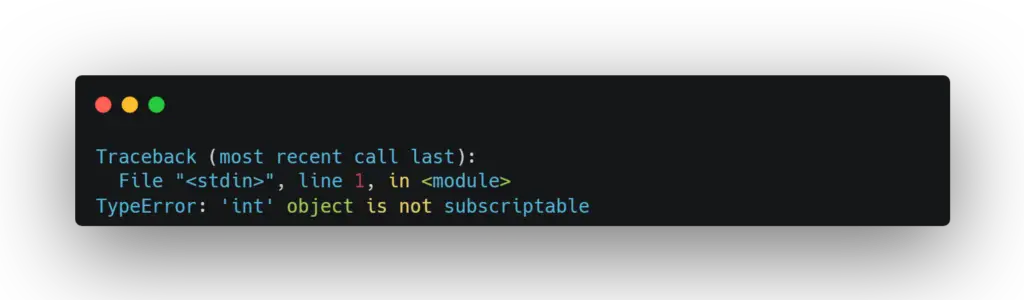
In this case, you can remove the square brackets, and the code will work as expected:
my_scalar = 5
print(my_scalar) # Prints 5 (without using an index)
Another common error while dealing with lists is using an index that is out of bounds for the list or array you are trying to access. For example, consider the following code:
my_list = [1, 2, 3]
print(my_list[3])
This will give an error because my_list only has three elements
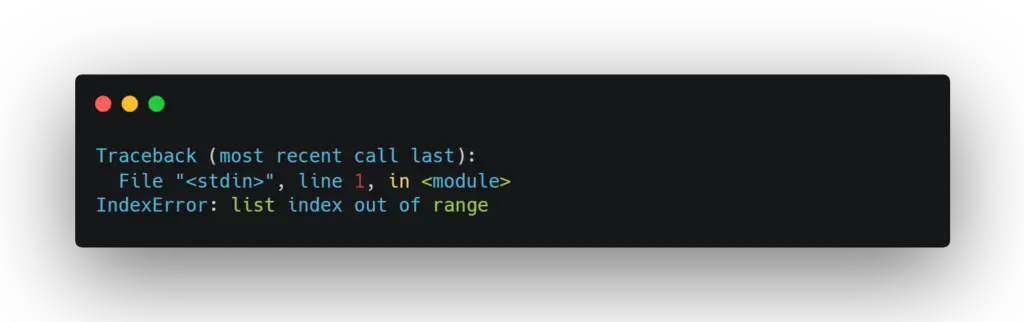
This code gives an error because we are trying to access the fourth element of my_list
(which has an index of 3), but our list only has three elements. To fix this, we need to ensure that we use an index within our list’s bounds.
How To Solve Python Invalid Index to Scalar Variable?
There are a few different ways to solve this error, depending on the cause. If you are accidentally trying to use an index on a scalar variable, you can remove the square brackets, and the code will work as expected:
my_scalar = 5
print(my_scalar) # Prints 5 (without using an index)
If you are using an index that is out of bounds for the list or array you are trying to access, you need to make sure that you are using an index that is within the bounds of your list. For example, if we want to print the third element of our my_list
list from the previous section; we would do it like this:
my_list = [1, 2, 3]
print(my_list[2]) # Prints 3 (which is the third element in our list)
Finally, if you are trying to use an index on a variable that is not an interable object, For example, if we want to use our my_list list from the previous section, we would do it like this:
my_list = [1, 2, 3]
print(my_list[0]) # Prints 1 (which is the first element in our list)
Conclusion
In this article, we have discussed the “invalid index to scalar variable” error in Python. We have seen this error and why it might occur in your code. Finally, we have provided some tips on how to solve it.
References
To learn more, please follow pythonclear