A function object is a first-class object that can be passed as an argument to other functions, or assigned to a variable. You can call a function by using the () operator.
One such error is the “TypeError: function object is not subscriptable”. This error can occur for several reasons and in this article, we will explore some of those reasons and how to fix them.
Contents
What Does Subscriptable Mean?
It means that the object implements the __getitem__()
method. In other words, it describes objects that are “containers”, meaning they contain other objects. This includes strings, lists, tuples, and dictionaries.
The subscript operator, which is square brackets, retrieves items from subscriptable objects like lists or tuples.
The following are the only built-ins that are subscriptable:
string: "foobar"[3] == "b"
tuple: (1,2,3,4)[3] == 4
list: [1,2,3,4][3] == 4
dict: {"a":1, "b":2, "c":3}["c"] == 3
What Causes the TypeError: function object is not subscriptable?
There are two main causes of this error: attempting to call a function with incorrect arguments, or using the wrong syntax when trying to access a dictionary key or list item.
Let’s look at each of these in turn.
Calling a Function with Incorrect Arguments
This is the most common cause of the “TypeError: function object is not subscriptable” error. It occurs when you try to call a function with more or fewer arguments than it expects.
Consider the following code:
def print_message(msg):
print(msg)
msg = "Hello world!"
print_message[0]
The print_message() function expects a single argument, which is the message to be printed. However, in the code above, we are attempting to call the function without any arguments.
This results in the following error:
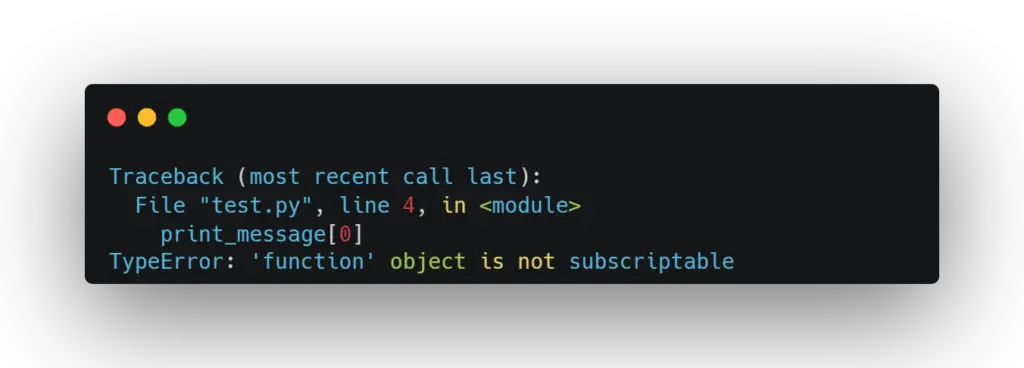
The reason this error occurs is that when you try to call a function without any arguments, Python will automatically try to subscribe to the function (hence the error message).
To fix this error, simply ensure that you are calling the function with the correct number of arguments. In our example above, we would need to provide a message argument when calling print_message():
def print_message(msg):
print(msg)
msg = "Hello world!"
print_message(msg) # Correct way to call function
Using Wrong Syntax When Accessing Dictionary Keys or List Items
Another common cause of this error is using incorrect syntax when trying to access a dictionary key or list item. For example, let’s say we have a list defined as follows:
my_list = [1, 2, 3]
And we want to access the second item on the list. The correct way to do this is with square brackets:
my_list = [1, 2, 3]
second_item = my_list[1] # Correct way to access 2nd item in list
However, if we try to access the second item using parentheses instead of square brackets, we get the following error:
File "test.py", line 4, in
second_item = my_list(1) # Incorrect way to access 2nd item in list
TypeError: 'function' object is not subscriptable
This is because when you use parentheses instead of square brackets, Python interprets this as trying to call the my_list() function with a single argument (in this case 1). Since my_list() is not a valid function, Python raises a TypeError.
To fix this error, simply use square brackets when accessing dictionary keys or list items. Do not use parentheses.
Conclusion
In this article, we looked at two main causes of the “TypeError: function object is not subscriptable” error in Python. We saw how this error can occur when trying to call a function with incorrect arguments or when using incorrect syntax when trying to access a dictionary key or list item.
To fix this error, simply ensure that you are using the correct number of arguments when calling a function and that you are using square brackets (not parentheses) when accessing dictionary keys or list items.
References
- Python Documentation
- StackOverflow
- StackOverflow – what-does-it-mean-if-a-python-object-is-subscriptable-or-not
For more such content follow python clear