Python’s import statement is one of the language’s most important features. It allows you to import modules from other parts of your code or external libraries. The import statement also imports variables and functions defined in other modules.
This article’ll explore how we import modules from the parent directory in Python (python import module from parent directory).
Contents
Using Import
When you use the import statement, Python looks for the module or package in a list of places. This list is called the Python path. The path is stored in the environment variable PYTHONPATH. If PYTHONPATH is not set, Python uses a default path that includes your current directory (.).
The import statement can be used with different syntaxes:
import module1 [ , module2 [ , ... ] ] from module1 import name1 [ , name2 [ , ... ] ] from module1 import *
The first form imports all names defined in module1 into the current namespace.
The second form imports only those names listed explicitly after from
. All other names are available but must be qualified with modname.
prefix, e.g., modname.name3
.
The third form causes all names defined in modname
to be imported except those beginning with _
(underscore).
In general, it’s more convenient to use the second form since it doesn’t pollute your namespace.
Ways in Python import module from parent directory.
When you import a module, the Python interpreter searches for the module in the following sequences –
1. The home directory of the program. 2. PYTHONPATH (an environment variable with a list of directories). 3. The default path for installed modules (on Windows it's C:\Python23\Lib , on Unix /usr/local/lib/python... ).
Python first checks whether the module is already loaded in sys.modules
. If this check fails, Python then searches each directory in sys.path
until it finds the module or raises an exception if it doesn’t find anything useful.
When a match is found and loaded, the search stops. If nothing is found during any phase of the search, ImportError
is raised: Not finding what you want but instead something similar to "No Module Named ..." indicates incorrect spelling (including case) or mispositioning of files relative to where you are executing your code from
A typical module search path might look like this on Windows:
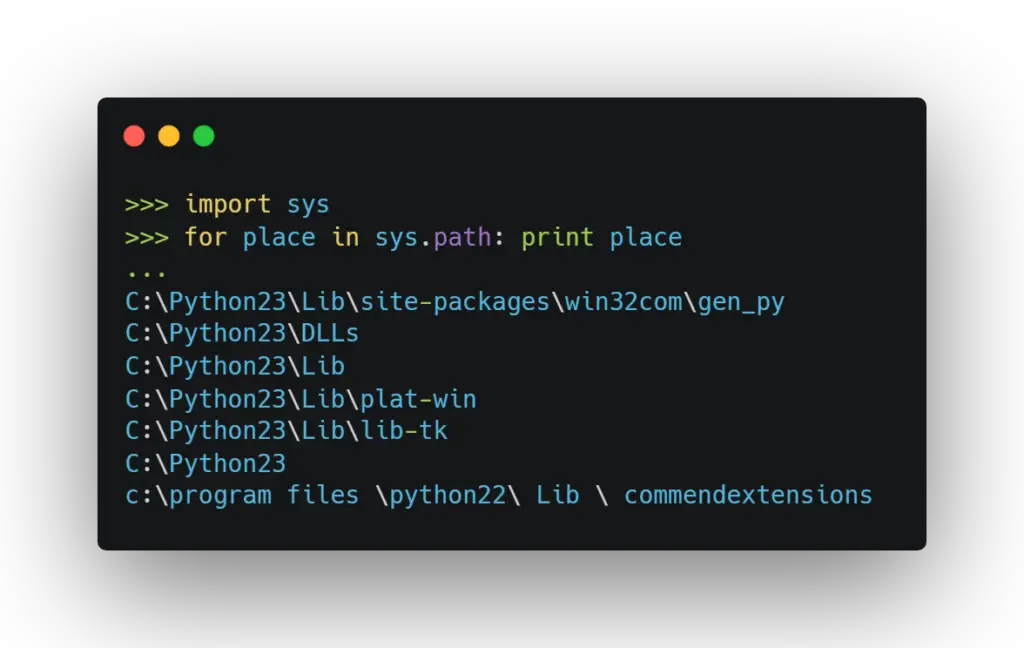
On UNIX, the default search path is often something like: /usr/local/lib/python:/usr/lib/python
. Of course, you can always add additional directories to sys.path
at runtime by appending them to the list, as follows:
import sys;
sys.path.append("/mydir")
This will make Python check that directory when it searches for modules, in addition to all the other places on sys.path
.
Let’s say you’ve written a module and placed it in C:\Python23\Lib\mymodule.py
. The module contains the following definitions:
def print_func( par ):
print "Hello : "
Now, you want to import this module into your Python program. Here is how you do it:
Importing Module from Parent Directory
In python import module from parent directory you can import the modules stored in the parent directory by adding the path of the parent directory to sys.path
variable of the system. You have to use the append method to add it instead of direct assignment as sys.path has some default paths already included, so if you will assign /home/desktop directly then all the default values get removed from sys.path . Let’s take an example where our scripts are located at /home/geeks, and we store our Python scripts inside the bin folder only, then for importing any module, we have to follow these steps –
Step 1: Add the path of parent directory (/home/geeks)
in front of sys.path
list like this-
>>>import sys #importing sys library for accessing sysyem variables like PYTHONPATH >>>sys.path.[0]="/home/geeks" #appending path of parent directory >>>print (sys.path) #checking sys.path list
Step 2: Now we store our scripts inside /home/geeks/bin
, so change your working directory to /home/geeks/bin
like this –
>>>import os >>>os.chdir("/home geeks / bin") #changing working directory to bin folder
Step 3: Create a test module name as “Addition
” with some simple addition function in it. Below is the code for the same-
# Addition Module def add(x,y): return x+y;
Our scripts are ready now, let’s see how we can use them now –
FileName : mainfile.py from Addition import add #importing Addition Module from bin folder print(add(10,20)) #calling add function form Addition Module and printing output Output : 30 #output
As we can see, in the first step we have appended the path of our parent directory which is /home/geeks
in front of sys.path
list, which makes it easy for the Python interpreter to search modules inside bin
folder too.
In the second step, we have changed our working directory /home/geeks/bin
so now Python will automatically search modules inside this folder also.
After that in the third step, we have created a module named Addition with a simple addition function and stored it inside bin
folder only. Next, through mainfile.py
script we are calling add function from Addition
Module and printing output which is coming as expected i.e., 30
.
This is a simple method for python import module from parent directory but what if your module is too long or has many sub-packages?
In such a case, it becomes a tedious task to append the path of each and every parent folder one by one like in the above example.
Another method – using Environment Variables.
One can use environment variables in python import module from parent directory. With this method, you can directly access any module stored anywhere in your system without changing PYTHONPATH manually every time –
By using Environment variables – you can set an environment variable named PYTHONPATH, whose value contains a list of directories. Any directory that you add to the PYTHONPATH environment variable will be searchable by the Python interpreter in addition to the default paths, sys.path
Let’s see how to do this –
>>>import os #importing OS library for calling environ function >>>print(os.environ['PYTHONPATH']) #environ is a dictionary which returns list of environment variables and their values Output : None
As we can see output is “None” because there is no environment variable set with name “PYTHONPATH” , let’s create it first-
For Linux/UNIX system-
$ export PYTHONPATH=/home/geeks:/usr/lib/python2.6:/usr/lib64/python2.6:/usr/local/lib64:~/.local:$XDG_DATA_DIRS
Verify whether the path has been set or not-
$ printenv | grep -i pythonpath (OR) $ env | grep -i pythonpath
For Windows System –
Go to Start -> Control Panel -> System -> Advanced tab -> Environment Variables.
Ensure that the PYTHONPATH system variable points to the directory where you want to store your Python files; if it doesn’t exist, create it. Append all directories that you need, each separated by a semicolon ( ; ). Close all windows and restart any applications, such as text editors or Integrated Development Environments (IDEs), so they will recognize the new environment variables.
Verify whether the path is set properly or not:
Go to command prompt and type-
>>>import sys
>>>sys.path
OR
$echo %PYTHONPATH% (On Windows system)
Once you have added a directory to PYTHONPATH , it’s searchable for future imports without modifying your code again. This way, python import module from parent directory can be easily done
Updating PYTHONPATH manually can get tedious if there are many directories, but luckily there are ways around this.
If you’re using UNIX/Linux/OS X, you can put export PYTHONPATH=/some/dir:/some/other/dir
in your ~/.bashrc
file will set up your environment automatically every time you log in and open a shell session. If you’re using Windows, see the Python documentation for information on how to set environment variables in the registry.
Conclusion
In this article, we have learned about different methods in python import module from parent directory. We also learned about setting up PYTHONPATH environment variable so that we can directly access any module stored anywhere in our system without changing PYTHONPATH manually every time.
References
You can also check out subscriptable objects, if your program can’t find __main module click here to see how to fix it.