The Python segmentation fault is a standard error that arises when a program tries to access a memory that can not be accessed, and this may occur when either the memory location is not accessible or the memory is only available for reading. This guide will give you a walkthrough of the Python segmentation fault and how it may occur and will provide the solutions to resolve these errors.
Contents
What is Python segmentation fault?
The Python segmentation fault is a type of IndexError caused when you try to access an inaccessible memory location or change or rewrite a memory only available to read. In this case, targeted memory is bound outside of an array. It may occur in various instances, for example, when you use the C extension to interact with the native libraries and codes or poorly written codes for the C extension or C++ extension. These cases may cause bugs in the program, which can cause the above mentioned IndexError.
How the Python segmentation fault is caused?
The Python segmentation fault IndexError can occur due to one of the many reasons mentioned below.
Trying to access an Invalid Memory Location
One of the main reasons for the Python segmentation fault IndexError is accessing an invalid memory location. As mentioned above, the above mentioned IndexError arises when you try to access a file or an object that is not present in an accessible memory location but instead in an inaccessible memory location or might not be present in the current memory location.
Syntax: –
import ctypes
# Load a shared library (e.g., a C library)
lib = ctypes.CDLL("libexample.so")
# Define a C function that triggers a segmentation fault
# This C function will try to access an invalid memory location
@ctypes.CFUNCTYPE(None)
def trigger_segfault():
invalid_pointer = ctypes.c_char_p(0) # An invalid memory location
invalid_pointer.value = 'A' # Attempt to write to the invalid memory location
# Call the C function to trigger the segmentation fault
trigger_segfault()
The above code will show the Python segmentation fault IndexError.
Using the C extension or C++ extension
Another reason for the Python segmentation fault IndexError is using the C or C++ extensions. For example, if you are using the NumPy library to create a large array but then attempt to write to an index that is present outside the array boundaries, then it will show the Python segmentation fault IndexError, as the NumPy library is present in the C extension and using its code to access the memory.
Syntax: –
import numpy as np
a = np.zeros((10000000,))
a[10000000] = 1
Due to limited memory
The Python segmentation fault IndexError can also be caused if limited memory is used. It is often caused when the program runs out of memory.
Syntax: –
def create_large_list():
lst = []
for i in range(10000000):
lst.append(i)
return lst
large_list = create_large_list()
If the above program is run on a machine with limited memory, the create_large_list() function will not work, the program will run out of memory, and the Python segmentation fault IndexError will arise.
How do we resolve the Python segmentation fault IndexError?
To solve the Python segmentation fault IndexError you can use one of the methods mentioned below.
Import fault handler
One of the methods for solving the Python segmentation fault IndexError is to import faulthandler. To use this method, you need to use the following set code before running the code.
Syntax: –
import faulthandler
faulthandler.enable()
python -Xfaulthandler my_program.py
Instead of the python -Xfaulthandler my_program.py, you can use the PYTHONFAULTHANDLER=1 python my_program.py in the code as an environmental.
Manage out-of-boundary array access
Another way to resolve the python segmentation fault IndexError is to ensure that boundary array is not being accessed.
Checking for System Library
Another way to resolve the Python segmentation fault IndexError is to make sure that the system libraries are installed. To check if the required system libraries are installed or not, you need to use the following set of commands. This command will show the list of all the present libraries in Python.
Syntax: –
$ ldd /path/to/python
Check the system files
Another way to resolve the Python segmentation fault IndexError is to update the system files. To check if the system files are up to date or not, you can use the following command.
Syntax:
$ sudo apt-get update && sudo apt-get upgrade -y
This command will update system files and add the missing files to the system.
FAQs
What is an array?
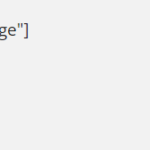
Array in Python are the unique variables that can hold multiple variables at a time.
Syntax: –
What is NumPy?
NumPy in Python stands for Numerical Python, a Python library used while working with arrays. It is also used with mathematical functions like linear algebra, matrices, and Fourier transform.
What is a faulthandler?
The faulthandler in Python is a module with functions that are used to dump tracebacks, specifically, on a fault on a user signal and on stack overflow. Usually, Python faulthandler is compatible with the system faulthandler like apport or Windows faulthandler.
Conclusion
This guide here has all the information that will help you to understand the Python segmentation fault IndexError and will also help you to resolve the error with the solutions listed above.
Reference
Follow us at PythonClear to learn more about solutions to general errors one may encounter while programming in Python.