The Typeerror: not supported between instances of str and int in Python, is a standard error that arises when a comparison operator is used between values of the “Str” type and “int” type. This type of comparison between two incompatible types always raises the TypeError.
This guide will help you find the causes and solutions for the Typeerror not supported between instances of str and int.
Contents
Difference between Str and Int
The “Str” and “Int” define two data types in Python. The “Str” represents the data type that can store characters. Whereas the “Int” data type stores the integers.
Usually, the “Str” data type contains non-numeric characters like alphabets, words, etc. It has more storage than the data storage for integers. Example: “Afgkj”
But in “Int” data type contains numerical data. The “Int” is always used for mathematical values. Generally, Integers are always positive whole numbers, and they are used in mathematical operations.
Since the “Int” and “Str” are two data types, they can not be used together, and if they are used, they will show the Typeerror: not supported between instances of “str” and “int”.
Solutions for Typeerror: not supported between instances of str and int
As mentioned above, the Typeerror: not supported between instances of str and int arises when the comparison operators differ. To resolve this error, the comparison operators must be the same type. So to do that, below is a list of ways you follow to convert the comparison operator into the same type and resolve the Typeerror: not supported between instances of “str” and “int”.
Converting String to an Integer
One way to resolve the Typeerror: not supported between instances of str and int is to convert the “Str” operator into the “Int” operator to make the data compatible. This can be done in two ways.
By using the “Int” class.
Syntax: –
my_str = '7'
my_int = 3
print(int(my_str) < my_int)
if int(my_str) < my_int:
print('my_str < my_int')
else:
print('my_str >= my_int')
The above example will show the result as false. Since the two can be compared now.
Another way to convert “Str” into “int” is to use “float()”. This can convert the Strings to floating point numbers. The floating numbers are easier to compare with the integer as they are more compatible with the integers than the strings.
Syntax: –
my_str = '6.8'
my_int = 4
print(float(my_str) < my_int)
The above example will show the result as false. Since the two can be compared now.
Converting Integer into a String
Similar to the conversion of strings into integers, Another way to resolve the Typeerror: not supported between instances of str and int is that integers can also be converted into Strings using the “input()” function, which can convert the integers into even numeric values.
Syntax:
from_input = input('2')
print(from_input)
print(type(from_input))
my_int = 6
print(int(from_input) <= my_int)
if int(from_input) <= my_int:
print('True')
The built-in input() function will convert the strings into valid integers to help compare. But if the integer used in the program is invalid, it may raise an error. You can handle the error using the ‘try’ and ‘except’ statements.
Try:
from_input = input('2')
print(from_input)
print(type(from_input))
my_int = 6
print(int(from_input) <= my_int)
except ValueError:
print('Invalid integer supplied')
Using a list Comprehension
Another solution for the Typeerror: not supported between instances of str and int, is using the List Comprehension. The List comprehensions are used if a list of strings needs to be converted into integers. List Comprehension is used by performing some operation for every element and converting the current item into integers.
For example, we try to find the highest and lowest number in a list.
Syntax: –
a_list = ['11', '12', 13, '30']
new_list = [int(x) for x in a_list]
print(new_list)
print(min(new_list))
print(max(new_list))
The above code will return the list’s highest and lowest values.
FAQ
What is List Comprehension?
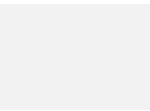
The List Comprehension is a statement that offers a shorter syntax based on the values of a pre-existing list to create a new list. This statement replaces the “for” statement with a conditional test.
What is an input() function?
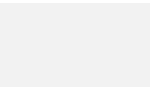
The input function is used for adding the input by the user.
What is float?
Float() is a type od reusable code or function that can convert the values into floating point numbers. The floating point numbers are decimal values or fractional numbers.
Syntax: –
x=float(18)
print(x)
What is str?
The str() is a function that is used to convert the specified values into a string.
What is Int?
The int() is a function that is used to convert the strings into an integer.
Conclusion
This guide can help you find the causes for Typeerror: not supported between instances of str and int and the probable solutions that can help you compatibly compare the “Str” and “Int” without any issues.
Reference
To learn more about some common errors follow Python Clear’s errors section.